Enhancing Python Applications with Tenacity: A Guide to Robust Retry Mechanisms
Takashi Yamamoto
Infrastructure Engineer · Leapcell
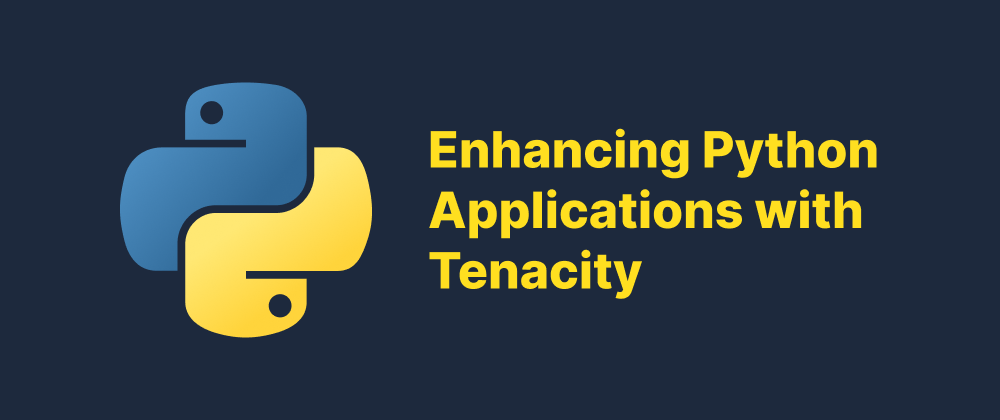
Key Takeaways
- Tenacity simplifies retry logic using a decorator-based approach.
- It supports extensive customization for stop and wait strategies.
- Using Tenacity improves application resilience against transient failures.
In the realm of software development, transient failures—such as network glitches, temporary service outages, or intermittent database issues—are common challenges. To build resilient applications that can gracefully handle these hiccups, implementing retry logic is essential. Python's Tenacity library offers a powerful and flexible solution for adding such retry mechanisms with minimal effort.
What Is Tenacity?
Tenacity is a general-purpose retrying library for Python, designed to simplify the process of adding retry behavior to functions and methods. It provides a decorator-based API that allows developers to specify retry conditions, wait strategies, and stop criteria in a clean and readable manner.
Key Features
- Decorator-Based API: Easily apply retry logic to functions using the
@retry
decorator. - Customizable Retry Conditions: Define which exceptions or return values should trigger a retry.
- Flexible Stop Strategies: Set conditions to stop retrying after a certain number of attempts or a specific time delay.
- Wait Strategies: Implement fixed, random, or exponential backoff waits between retries.
- Support for Async Functions: Apply retry logic to asynchronous functions seamlessly.
- Integration with Logging: Log retry attempts for better observability and debugging.
Installation
To install Tenacity, use pip:
pip install tenacity
Basic Usage
Here's how to use Tenacity to retry a function that may fail intermittently:
import random from tenacity import retry @retry def unreliable_function(): if random.random() < 0.7: raise Exception("Random failure occurred") return "Success!" print(unreliable_function())
In this example, unreliable_function
has a 70% chance of raising an exception. The @retry
decorator will automatically retry the function until it succeeds.
Advanced Configuration
Stopping After a Certain Number of Attempts
from tenacity import retry, stop_after_attempt @retry(stop=stop_after_attempt(5)) def limited_retries_function(): # Function logic here pass
Waiting Between Retries
from tenacity import retry, wait_fixed @retry(wait=wait_fixed(2)) def wait_between_retries_function(): # Function logic here pass
Combining Stop and Wait Strategies
from tenacity import retry, stop_after_attempt, wait_exponential @retry(stop=stop_after_attempt(5), wait=wait_exponential(multiplier=1, min=1, max=10)) def combined_strategy_function(): # Function logic here pass
Retrying on Specific Exceptions
from tenacity import retry, retry_if_exception_type @retry(retry=retry_if_exception_type(ValueError)) def specific_exception_function(): # Function logic here pass
Using Custom Retry Conditions
from tenacity import retry, retry_if_result def is_none(result): return result is None @retry(retry=retry_if_result(is_none)) def custom_condition_function(): # Function logic here return None # This will trigger a retry
Best Practices
- Avoid Infinite Retries: Always set a stop condition to prevent endless retry loops.
- Implement Backoff Strategies: Use exponential backoff to reduce load on external services during retries.
- Log Retry Attempts: Integrate logging to monitor retry behavior and diagnose issues effectively.
- Handle Exceptions Gracefully: Ensure that exceptions are properly caught and handled to maintain application stability.
Conclusion
Tenacity provides a robust and flexible framework for implementing retry logic in Python applications. By leveraging its features, developers can enhance the resilience of their applications, ensuring they can withstand transient failures gracefully. Whether dealing with unreliable network calls, flaky APIs, or intermittent database connections, Tenacity offers a clean and efficient solution to keep your applications running smoothly.
For more detailed information and advanced usage, refer to the official Tenacity documentation.
FAQs
Tenacity is used to implement retry logic for functions that may fail due to transient errors.
Yes, Tenacity fully supports retrying asynchronous functions.
Use stop_after_attempt(n)
to cap retry attempts.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ