Understanding `defaultdict` in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
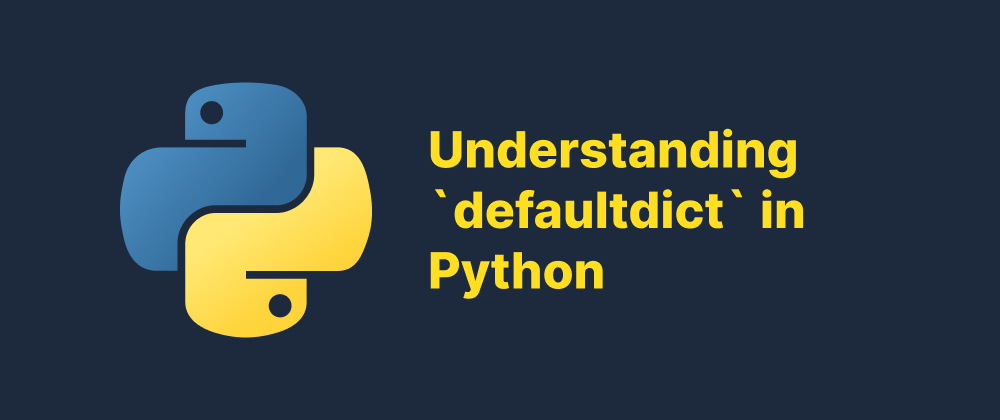
Key Takeaways
defaultdict
automatically initializes missing keys with a default value.- It simplifies common tasks like counting and grouping in dictionaries.
- A factory function must be provided to define default values.
When working with dictionaries in Python, one common issue developers face is dealing with missing keys. Accessing a key that doesn't exist in a standard dictionary (dict
) will raise a KeyError
. This is where collections.defaultdict
comes in — a powerful alternative that simplifies dictionary operations by providing default values for nonexistent keys.
What is defaultdict
?
defaultdict
is a subclass of the built-in dict
class. It overrides one method — __missing__
— to return a default value for missing keys instead of throwing an error. It is part of Python’s collections
module and requires importing before use.
from collections import defaultdict
Basic Usage
To create a defaultdict
, you must provide a factory function — a callable that returns the default value each time a missing key is accessed.
from collections import defaultdict # A defaultdict with int will return 0 for missing keys counts = defaultdict(int) counts['apple'] += 1 print(counts) # Output: defaultdict(<class 'int'>, {'apple': 1})
In the above example, int()
returns 0
, so the first access to 'apple'
doesn't raise a KeyError
— instead, it initializes with 0
, then adds 1
.
Common Use Cases
Counting Elements
defaultdict(int)
is frequently used for counting occurrences of items:
words = ['apple', 'banana', 'apple', 'orange', 'banana', 'apple'] counter = defaultdict(int) for word in words: counter[word] += 1 print(counter) # Output: defaultdict(<class 'int'>, {'apple': 3, 'banana': 2, 'orange': 1})
Grouping Data
defaultdict(list)
is useful for grouping items into lists:
from collections import defaultdict students = [ ('Math', 'Alice'), ('Math', 'Bob'), ('English', 'Alice'), ('Science', 'Charlie') ] grouped = defaultdict(list) for subject, name in students: grouped[subject].append(name) print(grouped) # Output: defaultdict(<class 'list'>, {'Math': ['Alice', 'Bob'], 'English': ['Alice'], 'Science': ['Charlie']})
Factory Functions
The factory can be any callable — not just built-ins like int
or list
. For example, you can use a lambda or custom function:
default_str = defaultdict(lambda: 'N/A') print(default_str['unknown']) # Output: N/A
Comparison with dict.get()
and setdefault()
While methods like dict.get(key, default)
or dict.setdefault(key, default)
can avoid KeyError
, defaultdict
is cleaner and more efficient when you need to repeatedly initialize default values.
Caveats
- All missing keys will be added to the dictionary once accessed, even if only for reading.
- If your default factory involves expensive operations, use with care — or consider lazy initialization.
Conclusion
defaultdict
is a highly useful tool in Python for simplifying dictionary usage when dealing with default values. Whether you're counting, grouping, or simply avoiding boilerplate initialization code, defaultdict
can make your code cleaner and more efficient.
For more complex use cases or deeply nested dictionaries, defaultdict
can also be nested itself:
tree = defaultdict(lambda: defaultdict(list)) tree['fruits']['red'].append('apple') print(tree)
Explore it — and it may become one of your favorite Python tools.
FAQs
The key is created and initialized using the factory function.
defaultdict
avoids repetitive code by initializing defaults automatically.
Yes, defaultdict
can be nested to build structures like trees or grouped mappings.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ