Understanding JSON Arrays: A Practical Guide
Wenhao Wang
Dev Intern · Leapcell
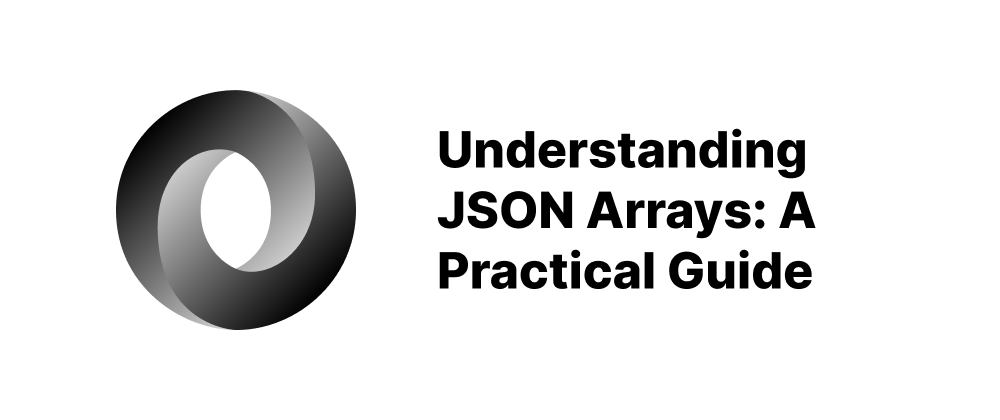
Key Takeaways
- JSON arrays are ordered lists that can contain various data types.
- Arrays are essential for structuring and transmitting collections of data.
- Proper parsing and formatting are crucial for effective JSON array use.
JSON (JavaScript Object Notation) is a lightweight data-interchange format that's easy for humans to read and write, and easy for machines to parse and generate. One of its fundamental structures is the array, which is widely used to represent ordered collections of data.
What Is a JSON Array?
A JSON array is an ordered list of values enclosed in square brackets []
. Each value is separated by a comma and can be of any valid JSON data type: string, number, object, array, boolean, or null. For example:
["apple", "banana", "cherry"]
This array contains three string elements. JSON arrays can also include mixed data types:
["text", 42, true, null, {"key": "value"}, [1, 2, 3]]
Key Characteristics
- Order Matters: Elements in a JSON array are ordered, and their position is significant.
- Zero-Based Indexing: The first element is at index 0.
- Mixed Types: Arrays can contain elements of different types, including nested arrays and objects.
Accessing JSON Array Elements
In programming languages like JavaScript, you can access elements of a JSON array using bracket notation:
let fruits = ["apple", "banana", "cherry"]; console.log(fruits[0]); // Outputs: apple
For nested arrays or objects:
let data = { "fruits": ["apple", "banana", "cherry"] }; console.log(data.fruits[1]); // Outputs: banana
Common Use Cases
- Lists of Items: Representing a collection of items, such as products, users, or messages.
- Nested Structures: Modeling complex data with arrays of objects or arrays within arrays.
- Data Exchange: Transmitting structured data between a server and a client in web applications.
Example: Array of Objects
[ { "name": "Alice", "age": 30 }, { "name": "Bob", "age": 25 } ]
This JSON array contains two objects, each representing a person with name
and age
properties.
Parsing and Stringifying JSON Arrays
In JavaScript, you can convert a JSON string to an object using JSON.parse()
and convert an object to a JSON string using JSON.stringify()
:
let jsonString = '[{"name":"Alice","age":30},{"name":"Bob","age":25}]'; let users = JSON.parse(jsonString); console.log(users[0].name); // Outputs: Alice let newJsonString = JSON.stringify(users); console.log(newJsonString);
Best Practices
- Consistent Data Types: While JSON arrays can hold mixed types, it's advisable to keep elements consistent for easier processing.
- Avoid Trailing Commas: JSON does not allow trailing commas after the last element in an array.
- Proper Formatting: Use tools or libraries to validate and format JSON data to ensure correctness.
Understanding JSON arrays is essential for working with structured data in modern web development. They provide a flexible way to represent and manipulate collections of data across various programming environments.
FAQs
Yes, JSON arrays can include strings, numbers, booleans, objects, arrays, and null.
Use zero-based indexing, e.g., array[0]
for the first element.
No, JSON syntax does not permit trailing commas.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ