Understanding Go's select: Concepts, Usage, and Best Practices
James Reed
Infrastructure Engineer · Leapcell
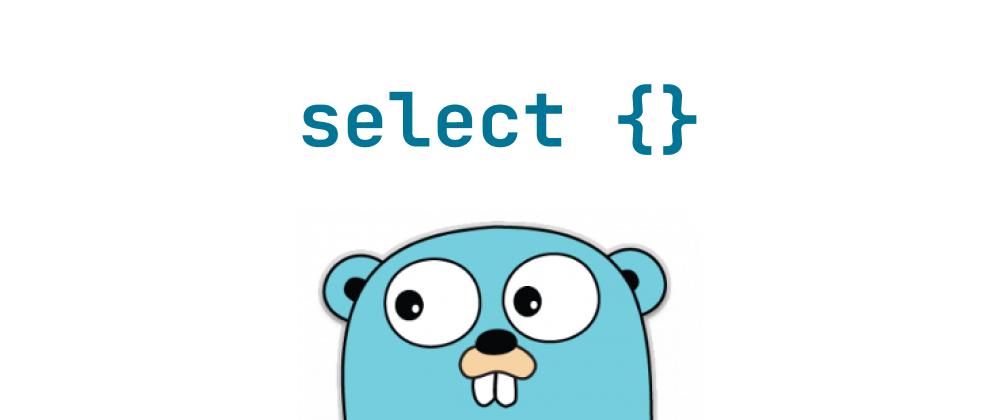
Basic Concepts
In Go, select
is a control structure used to handle multiple channel operations. It is very powerful and is often used in concurrent programming, especially when you need to select an available operation from multiple channels. Below is a detailed explanation of how to use select
and some common scenarios:
Basic Syntax
select { case <-ch1: // Code executed when ch1 is readable case ch2 <- value: // Code executed when ch2 is writable case result := <-ch3: // Read data from ch3 and assign to result default: // Code executed if no case is ready }
The working principle of select
is similar to switch
, but it is specifically designed for channel operations. It blocks and waits until one of the cases' channel operations can be executed. If multiple cases are ready at the same time, select
will randomly choose one to execute. If no case is ready and there is a default
, the default
branch will be executed.
Usage Scenarios and Examples
Reading Data from Multiple Channels
When you need to read data from multiple channels, you can use select
to avoid being blocked on a single channel.
package main import ( "fmt" "time" ) func main() { ch1 := make(chan string) ch2 := make(chan string) go func() { time.Sleep(1 * time.Second) ch1 <- "data1" }() go func() { time.Sleep(2 * time.Second) ch2 <- "data2" }() for i := 0; i < 2; i++ { select { case msg1 := <-ch1: fmt.Println("Received from ch1:", msg1) case msg2 := <-ch2: fmt.Println("Received from ch2:", msg2) } } }
Output:
Received from ch1: data1
Received from ch2: data2
In this example, select
waits for data from either ch1
or ch2
, and executes the corresponding case depending on which channel has data first.
Sending Data to Multiple Channels
You can also use select
to decide which channel to send data to.
package main import "fmt" func main() { ch1 := make(chan int) ch2 := make(chan int) go func() { for { select { case ch1 <- 1: fmt.Println("Sent to ch1") case ch2 <- 2: fmt.Println("Sent to ch2") } } }() time.Sleep(1 * time.Second) }
In this example, select
tries to send data to ch1
or ch2
, depending on which channel is ready to receive.
Using Default to Handle No Ready Channel
If no channel is ready, select
will block. To avoid blocking, you can add a default
branch.
package main import ( "fmt" "time" ) func main() { ch := make(chan string) select { case msg := <-ch: fmt.Println("Received:", msg) default: fmt.Println("No data, skipping") } go func() { time.Sleep(1 * time.Second) ch <- "delayed data" }() time.Sleep(2 * time.Second) msg := <-ch fmt.Println("Received:", msg) }
Output:
No data, skipping
Received: delayed data
The default
branch allows select
to execute immediately when no channel is ready, thus avoiding blocking.
Combining time.After to Implement Timeouts
select
is often used with time.After
to implement timeout mechanisms.
package main import ( "fmt" "time" ) func main() { ch := make(chan string) go func() { time.Sleep(2 * time.Second) ch <- "operation completed" }() select { case msg := <-ch: fmt.Println(msg) case <-time.After(1 * time.Second): fmt.Println("timeout") } }
Output:
timeout
In this example, if ch
does not receive data within 1 second, time.After
triggers the timeout logic.
Empty Select for Permanent Blocking
An empty select
will block forever, and is usually used for making the main goroutine wait for other goroutines to complete.
package main func main() { select {} }
This will cause the program to block indefinitely, and is often combined with for
loops or other logic.
Points to Note
- Randomness: If multiple cases are ready at the same time,
select
will randomly choose one to execute. It will not favor any particular channel. - Blocking: Without a
default
,select
will block until one of the cases is ready. - Uninitialized Channels: If a channel is
nil
, its corresponding case will never be selected. - Deadlock Risk: If none of the channels are operable and there is no
default
, this will lead to a deadlock.
Summary
- Use
select
to handle reading and writing operations on multiple channels. - Use
default
to avoid unnecessary blocking. - Combine with
time.After
to implement timeout control. - Pay attention to channel states to avoid deadlocks.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ