Rust Fundamentals Through 24 Minimal Examples
James Reed
Infrastructure Engineer · Leapcell
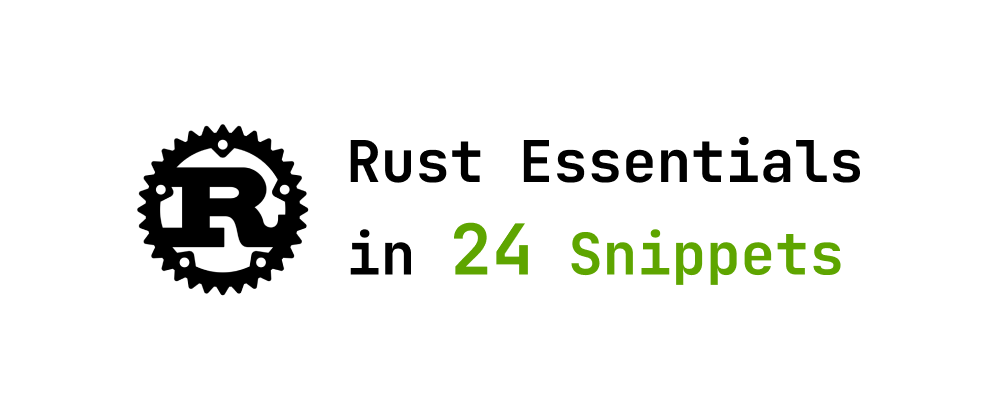
Rust is a systems programming language renowned for its safety, performance, and concurrency, featuring a rich and unique set of language constructs. This article introduces Rust's core capabilities through 24 concise code examples.
Pattern Matching
Rust’s match
expression implements pattern matching, allowing you to check whether a value fits a series of patterns.
let number = Some(42); match number { Some(x) => println!("The number is {}", x), None => println!("There is no number"), }
This code demonstrates how to use the match
expression to handle different cases of the Option
type.
Ownership and Lifetimes
With lifetime parameters, Rust ensures that references remain valid and avoids dangling pointers.
fn longest<'a>(x: &'a str, y: &'a str) -> &'a str { if x.len() > y.len() { x } else { y } }
This function takes two string slices and returns the longer one. The lifetime 'a
guarantees that the returned reference is valid.
Generics
Generics allow functions and structs to be defined without specifying exact types.
fn largest<T: PartialOrd + Copy>(list: &[T]) -> T { list.iter().cloned().max_by(|a, b| a.partial_cmp(b).unwrap()).unwrap() }
This generic function largest
finds the maximum value in a list of any type that implements the PartialOrd
and Copy
traits.
Traits
Traits are similar to interfaces, defining a set of methods that can be implemented.
trait Summary { fn summarize(&self) -> String; }
The Summary
trait can be implemented by any type to provide a textual summary of the object.
Type Casting
Rust provides several ways to perform type conversions.
let decimal: f64 = 6.0; let integer: i32 = decimal as i32; // Explicit type conversion
This code shows how to explicitly convert a value of type f64
to i32
.
Error Handling
Rust uses the Result
type to handle potential errors.
fn divide(a: f64, b: f64) -> Result<f64, String> { if b == 0.0 { Err("Cannot divide by zero".to_string()) } else { Ok(a / b) } }
This divide
function returns an error when the divisor is zero.
Iterators
Iterators are a powerful abstraction in Rust for handling sequences.
let a = [1, 2, 3]; let mut iter = a.iter(); while let Some(&item) = iter.next() { println!("{}", item); }
This code traverses an array using an iterator.
Closures
Closures are anonymous functions in Rust.
let list = vec![1, 2, 3]; let even_numbers: Vec<i32> = list.into_iter().filter(|&x| x % 2 == 0).collect();
Here, a closure is used to filter out even numbers from a Vec
.
Async Programming
Asynchronous code allows a program to perform other tasks while waiting for I/O operations.
async fn fetch_data() -> Result<(), Error> { // Code to fetch data asynchronously Ok(()) }
This fetch_data
function is asynchronous and can be called within an async runtime.
Smart Pointers
Smart pointers are pointers with additional capabilities and dynamic behavior.
use std::rc::Rc; let a = Rc::new(5); let b = Rc::clone(&a);
Here, Rc<T>
is a reference-counted smart pointer, allowing multiple owners of the same data.
Threads
Rust's std::thread
module provides capabilities to create and manage threads.
use std::thread; let handle = thread::spawn(|| { println!("Hello from a thread!"); }); handle.join().unwrap();
This code spawns a new thread and prints a message within it.
Channels
Channels are a mechanism in Rust for communication between threads.
use std::sync::mpsc; let (tx, rx) = mpsc::channel(); tx.send("Hello").unwrap(); let message = rx.recv().unwrap();
A channel is created here to pass messages between threads.
Atomic Types
Atomic types provide thread-safe shared state.
use std::sync::atomic::{AtomicUsize, Ordering}; let count = AtomicUsize::new(0);
AtomicUsize
is an unsigned integer that can be safely used in multi-threaded contexts.
Conditional Compilation
Conditional compilation allows different code to be compiled depending on the platform or configuration.
#[cfg(target_os = "windows")] fn is_windows() -> bool { true }
This attribute macro enables the is_windows
function only when the target operating system is Windows.
Macros
Macros are a powerful feature in Rust that allow code to generate code.
#[macro_use] extern crate serde_derive;
Here, the serde_derive
macro simplifies the writing of serialization and deserialization code.
Modules and Packages
The module system allows organizing code into a hierarchical structure.
mod my_module { pub fn do_something() { // ... } }
my_module
is a module within the current file and contains a function do_something
that can be accessed from outside.
Feature Gates
Feature gates are a way to prevent certain features from being misused.
#![feature(untagged_unions)]
This attribute macro enables the experimental untagged_unions
feature that is not yet stable in Rust.
Memory Allocation
Rust allows customization of memory allocators.
use std::alloc::{GlobalAlloc, Layout}; struct MyAllocator; unsafe impl GlobalAlloc for MyAllocator { unsafe fn alloc(&self, _layout: Layout) -> *mut u8 { // ... } }
Here, a custom global allocator MyAllocator
is defined.
Raw Pointers
Raw pointers offer low-level memory control.
let mut v = vec![1, 2, 3]; let ptr: *mut i32 = v.as_mut_ptr();
This code gets a raw pointer to a Vec
, allowing direct manipulation of its internal integers.
Unions
Unions allow different data types to share memory.
union MyUnion { i: i32, f: f32, }
MyUnion
can store either an i32
or an f32
, but only one at a time.
Enums
Enums in Rust are used to represent a type that can be one of several variants.
enum Message { Quit, Move { x: i32, y: i32 }, Write(String), }
The Message
enum can be Quit
, a Move
with coordinates, or a Write
containing a string.
Destructuring
Destructuring allows extracting values from structs or tuples.
let (x, y, z) = (1, 2, 3);
This destructures the tuple to simultaneously create three variables.
Lifetime Elision
Rust’s compiler can automatically infer lifetimes in certain cases.
fn borrow<'a>(x: &'a i32, y: &'a i32) -> &'a i32 { if *x > *y { x } else { y } }
In this function, the compiler can automatically infer the lifetime parameters.
Inline Assembly
Inline assembly allows embedding assembly instructions within Rust code.
// Requires specific architecture and environment setup unsafe { asm!("nop", options(nomem, nostack)); }
This code uses the asm!
macro to insert a nop
(no operation) instruction.
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ