Practical Guide to Pipeline Pattern in Go
James Reed
Infrastructure Engineer · Leapcell
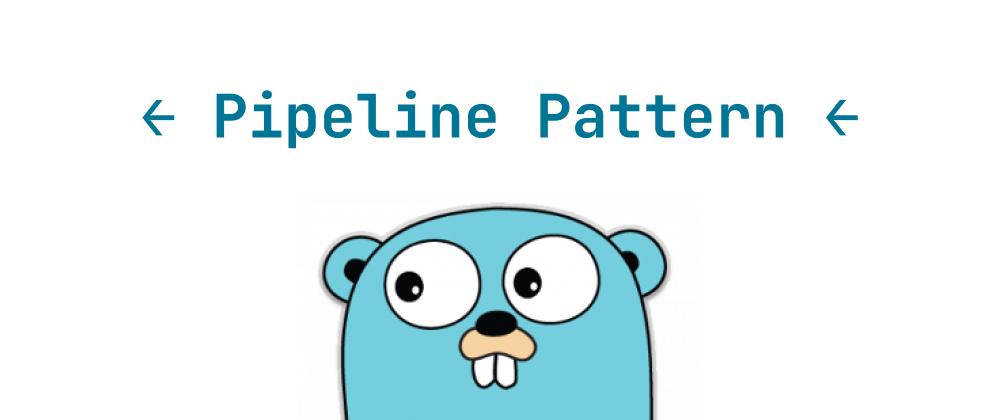
The Pipeline Design Pattern is a design pattern commonly used for data stream processing, allowing data to flow between different processing units to form a data processing pipeline.
The Go language, with its native support for goroutines and channels, is naturally suited for implementing the pipeline pattern, especially when handling concurrent tasks and data streams. The core idea of the pipeline pattern is to break the data processing process into multiple steps, each connected by a pipeline, forming a flexible streaming processing system.
This article will provide a detailed introduction to the implementation principles, best practices, and application scenarios of the pipeline design pattern in Go.
Overview of the Pipeline Design Pattern
The pipeline design pattern is typically used to transfer a data stream from one processing unit to the next. Each processing unit (or stage) is responsible for performing a specific operation and then passing the result to the next unit. This design pattern is especially suitable for scenarios that require multi-stage processing, concurrent control, or efficient resource management.
The pipeline design pattern has the following characteristics:
- Stage-wise Processing: The data stream passes through multiple stages, each responsible for an individual task.
- Concurrent Execution: Each stage can usually be executed concurrently and independently, utilizing Go’s goroutines to improve processing efficiency.
- Decoupling: Each stage only focuses on its own processing logic and does not need to know about the implementation of other stages, making the system highly scalable and maintainable.
Implementation of Pipelines in Go
In Go, pipelines are usually implemented using goroutines and channels. Goroutines provide lightweight thread support, while channels offer a mechanism for data transfer and synchronization, making the implementation of the pipeline pattern more natural and efficient.
Basic Pipeline Implementation
The basic idea of Go’s pipeline pattern is: each stage (or processing unit) is connected by a channel, and data flows from one stage to another. Each stage is an independent goroutine that passes data through channels.
Example: Basic Pipeline Design
package main import ( "fmt" "time" ) // First stage: Generate data func generateData(ch chan<- int) { for i := 1; i <= 5; i++ { ch <- i time.Sleep(100 * time.Millisecond) // Simulate processing delay } close(ch) } // Second stage: Process data func processData(input <-chan int, output chan<- int) { for data := range input { output <- data * 2 // Multiply data by 2 } close(output) } // Third stage: Consume data func consumeData(ch <-chan int) { for data := range ch { fmt.Println("Processed data:", data) } } func main() { dataCh := make(chan int) processedCh := make(chan int) // Start each stage go generateData(dataCh) go processData(dataCh, processedCh) consumeData(processedCh) }
How the Pipeline Works
- Data Generation Stage: The
generateData
function generates data and sends it to the next stage via thedataCh
channel. - Data Processing Stage: The
processData
function receives data fromdataCh
, processes it (e.g., multiplies it by 2), and then passes it to the next stage viaprocessedCh
. - Data Consumption Stage: The
consumeData
function receives the processed data fromprocessedCh
and outputs it.
In this way, data flows between multiple processing stages, achieving a complete pipeline processing flow.
Concurrent Execution
In the example above, the generateData
and processData
stages are executed concurrently, with each stage running in its own goroutine. They are connected via channels, ensuring data can be safely passed between stages.
Extension of the Pipeline Pattern: Multi-Stage Pipelines
As requirements grow, pipelines can have more stages, each possibly involving different task processing. The pipeline pattern is highly suitable for such situations, as it allows multiple concurrent processing units to operate sequentially, with each unit able to scale independently.
Example: Multi-Stage Pipeline Design
package main import ( "fmt" "time" ) func stage1(ch chan<- int) { for i := 1; i <= 5; i++ { ch <- i time.Sleep(100 * time.Millisecond) } close(ch) } func stage2(input <-chan int, output chan<- int) { for val := range input { output <- val * 10 } close(output) } func stage3(input <-chan int) { for val := range input { fmt.Printf("Final result: %d\n", val) } } func main() { ch1 := make(chan int) ch2 := make(chan int) // Start each stage go stage1(ch1) go stage2(ch1, ch2) stage3(ch2) }
Error Handling and Callbacks in Pipelines
In real-world applications, errors may occur at certain processing stages in the data stream. Therefore, it is advisable to incorporate error handling into each stage of the pipeline. Error propagation can be achieved by returning errors from each stage and passing them to subsequent stages.
Gracefully Closing Pipelines
In Go, a channel is a finite data structure; once closed, no more data can be written to it. Thus, after processing data at each stage, it is important to close the pipeline to notify other stages to stop reading.
Application Scenarios for the Pipeline Design Pattern
The pipeline design pattern is very common in scenarios involving concurrent tasks, streaming data, task queues, and more. Here are some typical application scenarios:
- Concurrent Data Processing: For example, in log processing systems or image processing systems, the pipeline pattern can be used to assign different processing tasks to different goroutines.
- Real-Time Data Streams: For instance, social media data analysis or real-time stock monitoring can be handled by processing large amounts of streaming data through the pipeline pattern.
- Task Queues: In background task scheduling systems, multiple tasks can be distributed and processed through different pipeline stages.
Summary
With Go’s pipeline design pattern, we can effectively manage concurrent tasks and data flows. By leveraging goroutines and channels, the pipeline pattern makes program structure clearer and more modular, while also improving code scalability. Through the combination of multiple processing stages, we can achieve complex concurrent data processing flows.
- Pipeline Pattern: Tasks are divided into multiple stages, with each stage able to process independently and concurrently.
- Concurrent Control: Concurrency is controlled through goroutines and channels, reducing resource waste.
- Flexible Extension: As requirements grow, new stages and features can be easily added to the pipeline.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ