Metaprogramming in JavaScript: A Deep Dive into Reflection and Symbols
Daniel Hayes
Full-Stack Engineer · Leapcell
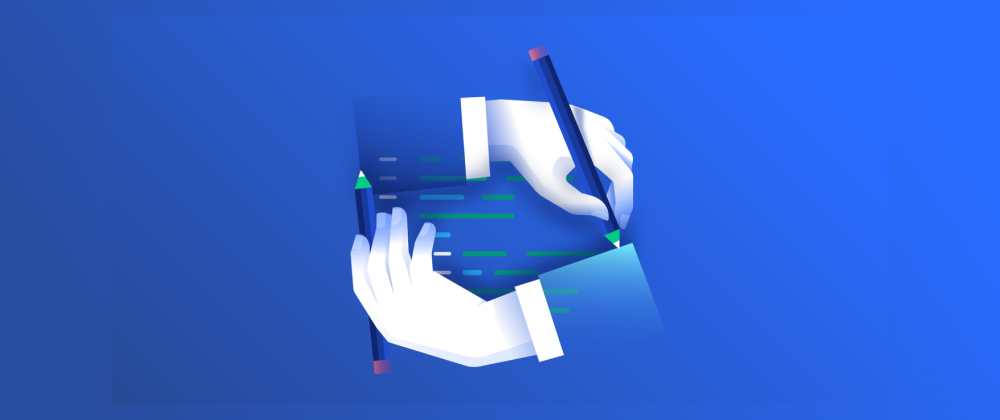
What is Reflection and Metaprogramming?
Let’s start with some theory—don’t worry, it won’t be too dry.
- Reflection: This refers to a program’s ability to inspect its own structure at runtime, such as examining an object’s properties or type. JavaScript provides the
Reflect
object, which contains a series of reflection methods that allow us to manipulate objects in a more elegant way. - Metaprogramming: This is a more advanced technique that allows us to write code that manipulates other code. In other words, you can write code to modify, intercept, or extend the behavior of other code. One powerful tool for metaprogramming in JavaScript is the
Proxy
.
Simply put, reflection allows us to "peek inside" the code, while metaprogramming lets us "control" the code’s behavior.
Reflection: Peeking Inside the Code
The Reflect
Object
Reflect
is a built-in object introduced in JavaScript that contains many useful methods for manipulating object properties, function calls, and more. Unlike some methods in Object
, Reflect
methods have consistent return values—if an operation fails, they return false
or undefined
instead of throwing an error.
Basic Reflection Operations:
const spaceship = { name: 'Apollo', speed: 10000, }; // Get property value console.log(Reflect.get(spaceship, 'name')); // 'Apollo' // Set property value Reflect.set(spaceship, 'speed', 20000); console.log(spaceship.speed); // 20000 // Check if property exists console.log(Reflect.has(spaceship, 'speed')); // true // Delete property Reflect.deleteProperty(spaceship, 'speed'); console.log(spaceship.speed); // undefined
Reflect
provides a more consistent and intuitive way to manipulate objects. Its design makes operations more controlled and avoids some of the pitfalls of traditional methods.
Defensive Programming for Object Operations
Sometimes, you may want to perform an operation on an object but are unsure if it will succeed. In such cases, Reflect
helps you write more defensive code.
function safeDeleteProperty(obj, prop) { if (Reflect.has(obj, prop)) { return Reflect.deleteProperty(obj, prop); } return false; } const spacecraft = { mission: 'Explore Mars' }; console.log(safeDeleteProperty(spacecraft, 'mission')); // true console.log(spacecraft.mission); // undefined console.log(safeDeleteProperty(spacecraft, 'nonExistentProp')); // false
With Reflect
, we can safely check and delete object properties without throwing errors.
Dynamic Method Invocation
In some advanced scenarios, you may need to dynamically call object methods, such as invoking a method based on a string name. Reflect.apply
is designed precisely for this situation.
const pilot = { name: 'Buzz Aldrin', fly: function (destination) { return `${this.name} is flying to ${destination}!`; }, }; const destination = 'Moon'; console.log(Reflect.apply(pilot.fly, pilot, [destination])); // 'Buzz Aldrin is flying to Moon!'
Reflect.apply
allows you to dynamically call methods without worrying about this
binding issues, making it very useful in dynamic scenarios.
Metaprogramming: Controlling Code Behavior
If reflection is about "peeking inside," then metaprogramming is about "controlling." In JavaScript, the Proxy
object is the key tool for metaprogramming. A Proxy
allows you to define custom behavior to intercept and redefine fundamental operations (such as property lookup, assignment, enumeration, and function calls).
Basic Usage of Proxy
A Proxy
takes two arguments:
- Target object: The object you want to proxy.
- Handler object: Defines "traps" (methods that intercept operations on the target).
const target = { message1: 'Hello', message2: 'World', }; const handler = { get: function (target, prop, receiver) { if (prop === 'message1') { return 'Proxy says Hi!'; } return Reflect.get(...arguments); }, }; const proxy = new Proxy(target, handler); console.log(proxy.message1); // 'Proxy says Hi!' console.log(proxy.message2); // 'World'
In this example, we intercepted the read operation for message1
and returned a custom message. Using Proxy
, we can easily change an object’s behavior without directly modifying the object itself.
Data Validation
Suppose you have an object storing user information, and you want to ensure that updates to user data follow specific rules. Proxy
can help enforce these rules.
const userValidator = { set: function (target, prop, value) { if (prop === 'age' && (typeof value !== 'number' || value <= 0)) { throw new Error('Age must be a positive number'); } if (prop === 'email' && !value.includes('@')) { throw new Error('Invalid email format'); } target[prop] = value; return true; }, }; const user = new Proxy({}, userValidator); try { user.age = 25; // Success user.email = 'example@domain.com'; // Success user.age = -5; // Throws an error } catch (error) { console.error(error.message); } try { user.email = 'invalid-email'; // Throws an error } catch (error) { console.error(error.message); }
With Proxy
, we can precisely control how properties are set, which is very useful in scenarios requiring strict data validation.
Observer Pattern
Suppose you have an object whose properties should trigger certain actions when modified, such as updating the UI or logging changes. Proxy
makes this easy to achieve.
const handler = { set(target, prop, value) { console.log(`Property ${prop} set to ${value}`); target[prop] = value; return true; }, }; const spaceship = new Proxy({ speed: 0 }, handler); spaceship.speed = 10000; // Console: Property speed set to 10000 spaceship.speed = 20000; // Console: Property speed set to 20000
Each time the speed
property of spaceship
is modified, we automatically log the change. This helps manage state in complex applications.
Defensive Programming
You may want to prevent certain object properties from being deleted or modified to ensure object integrity. Using Proxy
, we can create read-only properties or fully immutable objects.
const secureHandler = { deleteProperty(target, prop) { throw new Error(`Property ${prop} cannot be deleted`); }, set(target, prop, value) { if (prop in target) { throw new Error(`Property ${prop} is read-only`); } target[prop] = value; return true; }, }; const secureObject = new Proxy({ name: 'Secret Document' }, secureHandler); try { delete secureObject.name; // Throws an error } catch (error) { console.error(error.message); } try { secureObject.name = 'Classified'; // Throws an error } catch (error) { console.error(error.message); }
This approach helps create more robust and secure objects, preventing accidental modifications to critical data.
Symbol: Mysterious and Unique Identifiers
So far, we have explored Reflection (Reflection) and Metaprogramming (Metaprogramming). However, there is another equally important concept in JavaScript—Symbol—which plays a key role in implementing private properties and metaprogramming. Let's dive deeper and see how they can be combined in real-world applications to create more secure and powerful code.
What is a Symbol?
Symbol
is a primitive data type introduced in ES6, and its most important characteristic is uniqueness. Each Symbol
value is unique, even if two Symbol
values have the same description, they are not equal.
const sym1 = Symbol('unique'); const sym2 = Symbol('unique'); console.log(sym1 === sym2); // false
Because of this uniqueness, Symbols are especially useful as object property keys, making them a great way to create private properties.
Using Symbol as a Private Property
In JavaScript, there are no truly private properties, but Symbol
provides a way to mimic private properties. By using Symbol
, we can add properties that won't be exposed through normal property enumeration.
const privateName = Symbol('name'); class Spaceship { constructor(name) { this[privateName] = name; // Use Symbol as a private property } getName() { return this[privateName]; } } const apollo = new Spaceship('Apollo'); console.log(apollo.getName()); // Apollo console.log(Object.keys(apollo)); // [] console.log(Object.getOwnPropertySymbols(apollo)); // [ Symbol(name) ]
In this example:
- The
privateName
property does not appear inObject.keys()
, making it hidden from normal iteration. - However, if needed, we can explicitly retrieve
Symbol
properties usingObject.getOwnPropertySymbols()
.
This makes Symbol
an effective way to create "private" properties in JavaScript.
Preventing Property Name Collisions
When working on large-scale projects or third-party libraries, different parts of the code might accidentally use the same property name, leading to unexpected conflicts. Symbol
helps prevent such conflicts.
const libraryProp = Symbol('libProperty'); const obj = { [libraryProp]: 'Library data', anotherProp: 'Some other data', }; console.log(obj[libraryProp]); // 'Library data'
Since Symbol
is unique, even if another developer defines a property with the same name, it won't override your property.
Using Symbol for Metaprogramming
Besides being useful for private properties, Symbol
also plays an important role in metaprogramming, especially through built-in Symbols like Symbol.iterator
and Symbol.toPrimitive
, which allow us to modify JavaScript's default behaviors.
Symbol.iterator and Custom Iterators
Symbol.iterator
is a built-in Symbol
used to define an iterator method for an object. When you use a for...of
loop on an object, JavaScript internally calls the object's Symbol.iterator
method.
const collection = { items: ['🚀', '🌕', '🛸'], [Symbol.iterator]: function* () { for (let item of this.items) { yield item; } }, }; for (let item of collection) { console.log(item); } // Output: // 🚀 // 🌕 // 🛸
By defining a custom iterator, we can control how an object is iterated, which is particularly useful for custom data structures.
Symbol.toPrimitive and Type Conversion
Another useful built-in Symbol
is Symbol.toPrimitive
, which allows us to define custom type conversion rules for an object.
Normally, when an object is used in a mathematical operation or string context, JavaScript tries to convert it to a primitive type using .toString()
or .valueOf()
. With Symbol.toPrimitive
, we can fine-tune this behavior.
const spaceship = { name: 'Apollo', speed: 10000, [Symbol.toPrimitive](hint) { switch (hint) { case 'string': return this.name; case 'number': return this.speed; default: return `Spaceship: ${this.name} traveling at ${this.speed} km/h`; } }, }; console.log(`${spaceship}`); // Apollo console.log(+spaceship); // 10000 console.log(spaceship + ''); // Spaceship: Apollo traveling at 10000 km/h
With Symbol.toPrimitive
, we can control how objects behave in different contexts.
Combining Reflection, Metaprogramming, and Symbol
Now that we understand Symbol
, let's see how we can combine it with Reflect
and Proxy
to build more advanced and flexible programs.
Using Proxy to Intercept Symbol Operations
Since Proxy
can intercept object operations, we can also intercept accesses to Symbol properties for additional control.
const secretSymbol = Symbol('secret'); const spaceship = { name: 'Apollo', [secretSymbol]: 'Classified data', }; const handler = { get: function (target, prop, receiver) { if (prop === secretSymbol) { return 'Access Denied!'; } return Reflect.get(...arguments); }, }; const proxy = new Proxy(spaceship, handler); console.log(proxy.name); // Apollo console.log(proxy[secretSymbol]); // Access Denied!
Here, we used Proxy
to intercept access to the secretSymbol
property and return 'Access Denied!'
, effectively hiding classified data.
Implementing Flexible Data Validation
By combining Symbol
and Proxy
, we can create a dynamic validation system where certain properties are marked with Symbol
and validated before being set.
const validateSymbol = Symbol('validate'); const handler = { set(target, prop, value) { if (prop === validateSymbol) { if (typeof value !== 'string' || value.length < 5) { throw new Error('Validation failed: String length must be at least 5 characters'); } } target[prop] = value; return true; }, }; const spaceship = new Proxy({}, handler); try { spaceship[validateSymbol] = 'abc'; // Throws an error } catch (error) { console.error(error.message); // Validation failed: String length must be at least 5 characters } spaceship[validateSymbol] = 'Apollo'; // Success
This method allows us to tag certain properties with a Symbol and enforce strict validation.
Conclusion: Integrating Reflection, Metaprogramming, and Symbol into Real-World Applications
Symbol
is a powerful and unique tool in JavaScript that:
- Helps create private properties.
- Prevents property name conflicts.
- Enhances custom behavior using built-in symbols like
Symbol.iterator
andSymbol.toPrimitive
.
When combined with Reflect
and Proxy
, Symbol
can be used to:
- Intercept property access for security.
- Validate data dynamically.
- Customize object behavior efficiently.
Final Thoughts
Next time you're developing a JavaScript application, consider integrating Reflection, Metaprogramming, and Symbol to make your code more secure, flexible, and maintainable!
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ