How to Store JSON Data in Session Storage in Angular
Grace Collins
Solutions Engineer · Leapcell
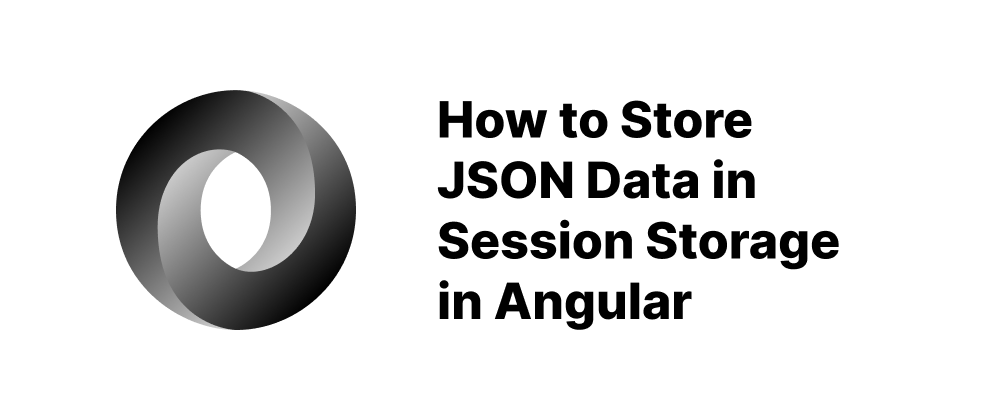
Key Takeaways
- JSON data must be stringified before storing in session storage.
- A dedicated Angular service improves code reuse and organization.
- Avoid storing sensitive data due to security risks.
Session storage is a powerful tool for temporarily storing data in a user's browser during a session. In Angular applications, effectively managing session storage can enhance user experience by preserving state across page reloads. This guide will walk you through storing and retrieving JSON data in session storage within an Angular context.([Stack Overflow][1])
Understanding Session Storage
Session storage is part of the Web Storage API, allowing you to store key-value pairs in a web browser. Unlike local storage, which persists data indefinitely, session storage retains data only for the duration of the page session. Once the browser tab is closed, the data is cleared.([Stack Abuse][2], [JavaScript in Plain English][3])
Storing JSON Data in Session Storage
Since session storage can only store strings, you'll need to serialize your JSON data before storing it. Here's how you can do it in an Angular component:([Medium][4])
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-example', template: `<p>Session Storage Example</p>` }) export class ExampleComponent implements OnInit { ngOnInit() { const user = { name: 'John Doe', email: 'john.doe@example.com' }; // Serialize and store the JSON object sessionStorage.setItem('user', JSON.stringify(user)); } }
In this example, the user
object is converted into a JSON string using JSON.stringify()
before being stored in session storage.([Stack Abuse][2])
Retrieving JSON Data from Session Storage
To retrieve and deserialize the JSON data from session storage:
const userData = sessionStorage.getItem('user'); if (userData) { const user = JSON.parse(userData); console.log(user.name); // Outputs: John Doe }
This code fetches the stringified JSON from session storage and parses it back into a JavaScript object using JSON.parse()
.([Stack Abuse][2])
Creating a Session Storage Service in Angular
For better code organization and reusability, consider creating a dedicated service to handle session storage operations:([Stack Overflow][5])
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class SessionStorageService { setItem(key: string, value: any): void { try { const serializedValue = JSON.stringify(value); sessionStorage.setItem(key, serializedValue); } catch (error) { console.error('Error saving to session storage', error); } } getItem<T>(key: string): T | null { try { const item = sessionStorage.getItem(key); return item ? JSON.parse(item) as T : null; } catch (error) { console.error('Error reading from session storage', error); return null; } } removeItem(key: string): void { sessionStorage.removeItem(key); } clear(): void { sessionStorage.clear(); } }
This service provides methods to set, get, remove, and clear items in session storage, handling serialization and deserialization internally.
Using the Session Storage Service
Inject the SessionStorageService
into your components to manage session storage:
import { Component, OnInit } from '@angular/core'; import { SessionStorageService } from './session-storage.service'; @Component({ selector: 'app-user', template: `<p>User Component</p>` }) export class UserComponent implements OnInit { constructor(private sessionStorageService: SessionStorageService) {} ngOnInit() { const user = { name: 'Jane Smith', email: 'jane.smith@example.com' }; this.sessionStorageService.setItem('user', user); const storedUser = this.sessionStorageService.getItem<{ name: string; email: string }>('user'); if (storedUser) { console.log(storedUser.name); // Outputs: Jane Smith } } }
This approach promotes cleaner code and separates concerns, making your application more maintainable.
Best Practices
- Error Handling: Always include try-catch blocks when parsing JSON to handle potential errors gracefully.
- Data Validation: Validate the data retrieved from session storage before using it to prevent unexpected behavior.
- Security Considerations: Avoid storing sensitive information in session storage, as it's accessible through browser developer tools.
- Service Abstraction: Encapsulate session storage logic within a service to promote code reuse and maintainability.
FAQs
Use JSON.stringify()
to convert the object to a string, then use sessionStorage.setItem()
.
It promotes clean, reusable, and maintainable code across components.
No, it’s accessible via browser tools, so avoid storing confidential information.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ