How to Escape Strings in JSON
Daniel Hayes
Full-Stack Engineer · Leapcell
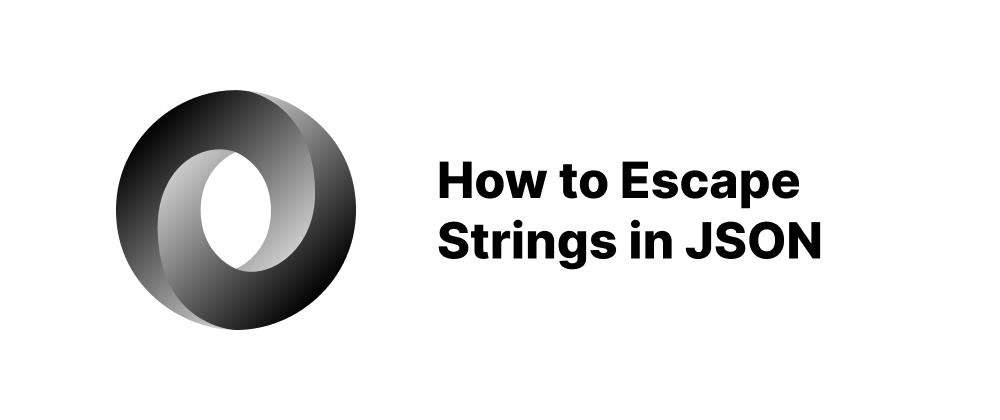
Key Takeaways
- JSON escaping is essential to avoid syntax errors and data corruption.
- Use built-in functions in programming languages to handle escaping automatically.
- Manual escaping should be avoided due to risk of mistakes.
JSON (JavaScript Object Notation) is a widely used data format for representing structured data. When working with JSON, it's crucial to ensure that strings are properly escaped to maintain data integrity and prevent parsing errors. This article delves into the essentials of JSON string escaping, common pitfalls, and best practices across various programming languages.
Why Escaping is Necessary in JSON
JSON strings must adhere to specific syntax rules. Certain characters within strings can disrupt the structure of JSON data if not properly escaped. These characters include:
- Double quotes (
"
): Used to delimit strings in JSON. - Backslashes (
\
): Escape character in JSON. - Control characters: Such as newline (
\n
), carriage return (\r
), tab (\t
), backspace (\b
), and form feed (\f
).
Failing to escape these characters can lead to:
- Parsing errors: Invalid JSON structure.
- Data corruption: Misinterpretation of string content.
- Security vulnerabilities: Potential for injection attacks.
JSON Escape Sequences
To safely include special characters in JSON strings, use the following escape sequences:
Character | Escape Sequence |
---|---|
" | \" |
\ | \\ |
/ | \/ |
Backspace | \b |
Form feed | \f |
Newline | \n |
Carriage return | \r |
Tab | \t |
Additionally, Unicode characters can be represented using the \uXXXX
format, where XXXX
is the four-digit hexadecimal code point. For example, the emoji 😊 can be represented as \uD83D\uDE0A
.
Escaping JSON Strings in Different Programming Languages
JavaScript
In JavaScript, the JSON.stringify()
method automatically escapes special characters:
const obj = { message: 'He said, "Hello, World!"\nNew line here.' }; const jsonString = JSON.stringify(obj); console.log(jsonString); // Output: {"message":"He said, \"Hello, World!\"\nNew line here."}
For manual escaping, a helper function can be used:
function escapeString(str) { return str .replace(/\\/g, '\\\\') .replace(/"/g, '\\"') .replace(/\n/g, '\\n') .replace(/\r/g, '\\r') .replace(/\t/g, '\\t'); } const escaped = escapeString('He said, "Hello, World!"\nNew line here.'); console.log(escaped); // Output: He said, \"Hello, World!\"\nNew line here.
Python
Python's json
module provides the dumps()
method to serialize objects, handling escaping automatically:
import json data = { "message": "He said, \"Hello, World!\"\nNew line here." } json_string = json.dumps(data) print(json_string) # Output: {"message": "He said, \"Hello, World!\"\nNew line here."}
Java
In Java, libraries like org.json
or Jackson
handle JSON serialization and escaping:
import org.json.JSONObject; public class Main { public static void main(String[] args) { JSONObject obj = new JSONObject(); obj.put("message", "He said, \"Hello, World!\"\nNew line here."); String jsonString = obj.toString(); System.out.println(jsonString); // Output: {"message":"He said, \"Hello, World!\"\nNew line here."} } }
PHP
PHP's json_encode()
function escapes special characters in strings:
<?php $data = array( "message" => "He said, \"Hello, World!\"\nNew line here." ); $jsonString = json_encode($data); echo $jsonString; // Output: {"message":"He said, \"Hello, World!\"\nNew line here."} ?>
Best Practices for JSON Escaping
-
Use Built-in Functions: Rely on language-specific functions like
JSON.stringify()
in JavaScript orjson.dumps()
in Python to handle escaping. -
Avoid Manual Escaping: Manual string escaping is error-prone. Utilize libraries and functions designed for JSON serialization.
-
Validate JSON Output: Use tools like JSONLint to validate your JSON data.
-
Be Cautious with User Input: Always sanitize and escape user-generated content to prevent injection attacks.
-
Handle Unicode Properly: When dealing with international characters, ensure they are correctly represented using Unicode escape sequences if necessary.
Conclusion
Properly escaping strings in JSON is vital for data integrity, application stability, and security. By leveraging built-in serialization methods and adhering to best practices, developers can ensure that their JSON data is correctly formatted and free from errors.
FAQs
Characters like quotes ("
), backslashes (\
), and control characters (e.g., \n
, \t
) must be escaped.
It's possible, but not recommended—use language-provided methods like JSON.stringify()
or json.dumps()
.
Unicode escaping ensures safe representation of characters outside the ASCII range.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ