Understanding File Globbing in Go
Grace Collins
Solutions Engineer · Leapcell
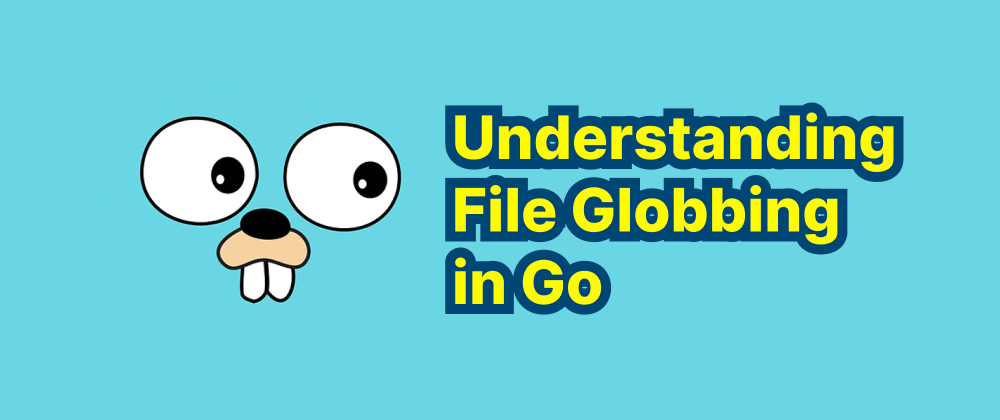
Key Takeaways
- Go's
filepath.Glob
enables pattern-based file matching using wildcards like*
and?
. - Proper handling of errors and empty matches is crucial when using
Glob
. - For advanced pattern matching, third-party packages like
gobwas/glob
can be used.
File globbing is a powerful mechanism used to match filenames or paths based on specific patterns. In Go, the path/filepath
package provides utilities to perform such operations, enabling developers to search for files and directories that match particular patterns.
What is Globbing?
Globbing refers to the process of using wildcard characters to define patterns for matching filenames or paths. Common wildcard characters include:
*
: Matches any sequence of non-separator characters (i.e., characters except for the path separator).?
: Matches any single non-separator character.[...]
: Matches any single character specified within the brackets. For example,[abc]
matches either 'a', 'b', or 'c'.
These patterns allow for flexible and efficient searching and matching of file paths.
Using filepath.Glob
in Go
The path/filepath
package's Glob
function is designed to return the names of all files matching a specified pattern. The syntax of the pattern adheres to the same rules as described above.
Here's the signature of the Glob
function:
func Glob(pattern string) (matches []string, err error)
pattern
: The glob pattern to match against file or directory names.matches
: A slice of strings containing the matched file or directory names.err
: An error value that reports any issues encountered while processing the pattern.
Example:
package main import ( "fmt" "log" "path/filepath" ) func main() { pattern := "*.go" matches, err := filepath.Glob(pattern) if err != nil { log.Fatal(err) } for _, match := range matches { fmt.Println(match) } }
In this example, the program searches for all files in the current directory that have a .go
extension and prints their names.
Important Considerations
-
Pattern Syntax: The pattern must be a valid glob pattern. If the pattern is malformed,
Glob
will return an error, specificallyfilepath.ErrBadPattern
. -
No Matches: If no files match the given pattern,
Glob
returns an empty slice and anil
error. It's essential to check the length of thematches
slice to determine if any matches were found. -
Absolute vs. Relative Paths: The
Glob
function can handle both absolute and relative patterns. Ensure that the pattern accurately reflects the desired search path. -
Cross-Platform Behavior: Be mindful of platform-specific path separators. While
/
is commonly used as the path separator in UNIX-like systems, Windows uses\
. Thepath/filepath
package accounts for these differences, but it's good practice to usefilepath.Join
and other utility functions to construct paths.
Advanced Globbing with Third-Party Packages
While the standard library's Glob
function is suitable for many use cases, there are scenarios where more advanced pattern matching is required, such as supporting patterns like {a,b}
for alternative matches. In such cases, third-party packages like github.com/gobwas/glob
can be utilized to enhance globbing capabilities.
Example using gobwas/glob
:
package main import ( "fmt" "log" "github.com/gobwas/glob" ) func main() { g, err := glob.Compile("{*.go,*.md}") if err != nil { log.Fatal(err) } files := []string{"main.go", "README.md", "example.txt"} for _, file := range files { if g.Match(file) { fmt.Println(file) } } }
In this example, the program uses the gobwas/glob
package to match files that have either a .go
or .md
extension. The Compile
function supports the {a,b}
syntax, allowing for more flexible pattern definitions.
Conclusion
File globbing is a valuable technique in file system operations, enabling pattern-based matching of file and directory names. Go's path/filepath
package provides the Glob
function to facilitate such operations with standard wildcard patterns. For more advanced globbing requirements, third-party packages like gobwas/glob
offer extended functionalities, allowing developers to implement complex pattern matching with ease.
FAQs
It returns an empty slice and a nil
error.
Yes, it supports both absolute and relative paths.
No, for that feature, use third-party libraries like gobwas/glob
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ