How To Check For Empty String In Golang
Grace Collins
Solutions Engineer · Leapcell
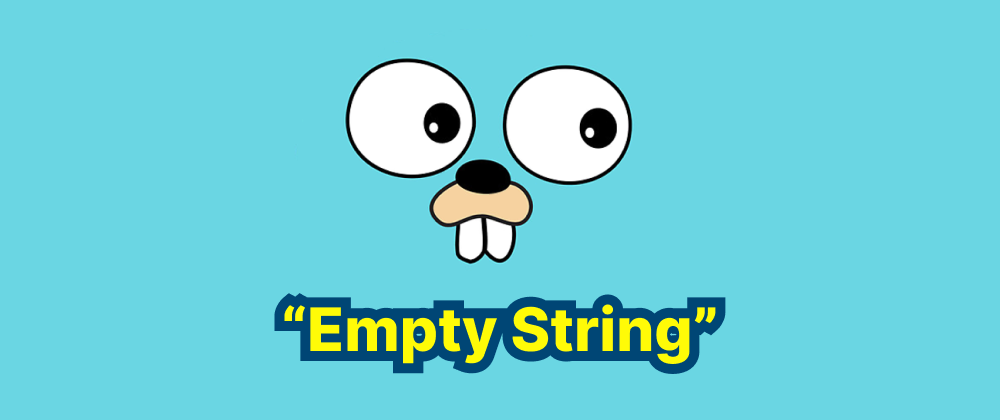
In Go, determining whether a string is empty is a common task that can be accomplished using two primary methods:
-
Direct Comparison with an Empty String Literal: This approach involves comparing the string directly to an empty string (
""
):if str == "" { // str is empty }
This method is straightforward and idiomatic in Go. It clearly conveys the intention of checking for an empty string.
-
Using the
len
Function: Alternatively, you can check the length of the string:if len(str) == 0 { // str is empty }
This method evaluates the length of the string and checks if it is zero.
Key Takeaways
- The preferred way to check for an empty string in Go is
str == ""
for clarity and readability. - Using
len(str) == 0
is an alternative but functionally equivalent approach. - To check for strings that contain only whitespace, use
strings.TrimSpace(str) == ""
.
Both methods are functionally equivalent and are optimized by the Go compiler to produce similar performance. The choice between them often comes down to personal or team preference. However, using str == ""
is generally preferred for its readability and clarity, as it directly expresses the intent to check for an empty string.
Handling Strings with Whitespace:
If you need to consider strings that contain only whitespace characters (such as spaces, tabs, or newlines) as empty, you can use the strings.TrimSpace
function from Go's standard library:
import "strings" if strings.TrimSpace(str) == "" { // str is empty or contains only whitespace }
The strings.TrimSpace
function removes all leading and trailing whitespace from the string. By comparing the result to an empty string, you can determine if the original string was either empty or consisted solely of whitespace characters.
FAQs
str == ""
is preferred for its readability and clarity.
No, both methods are functionally equivalent in Go.
Use strings.TrimSpace(str) == ""
to remove leading and trailing whitespace.
In summary, while both str == ""
and len(str) == 0
are valid methods to check for an empty string in Go, the former is often favored for its clarity. When dealing with strings that may contain only whitespace, incorporating strings.TrimSpace
ensures a more comprehensive check.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ