Go Dependency Management with Modules: Everything You Need to Know
James Reed
Infrastructure Engineer · Leapcell
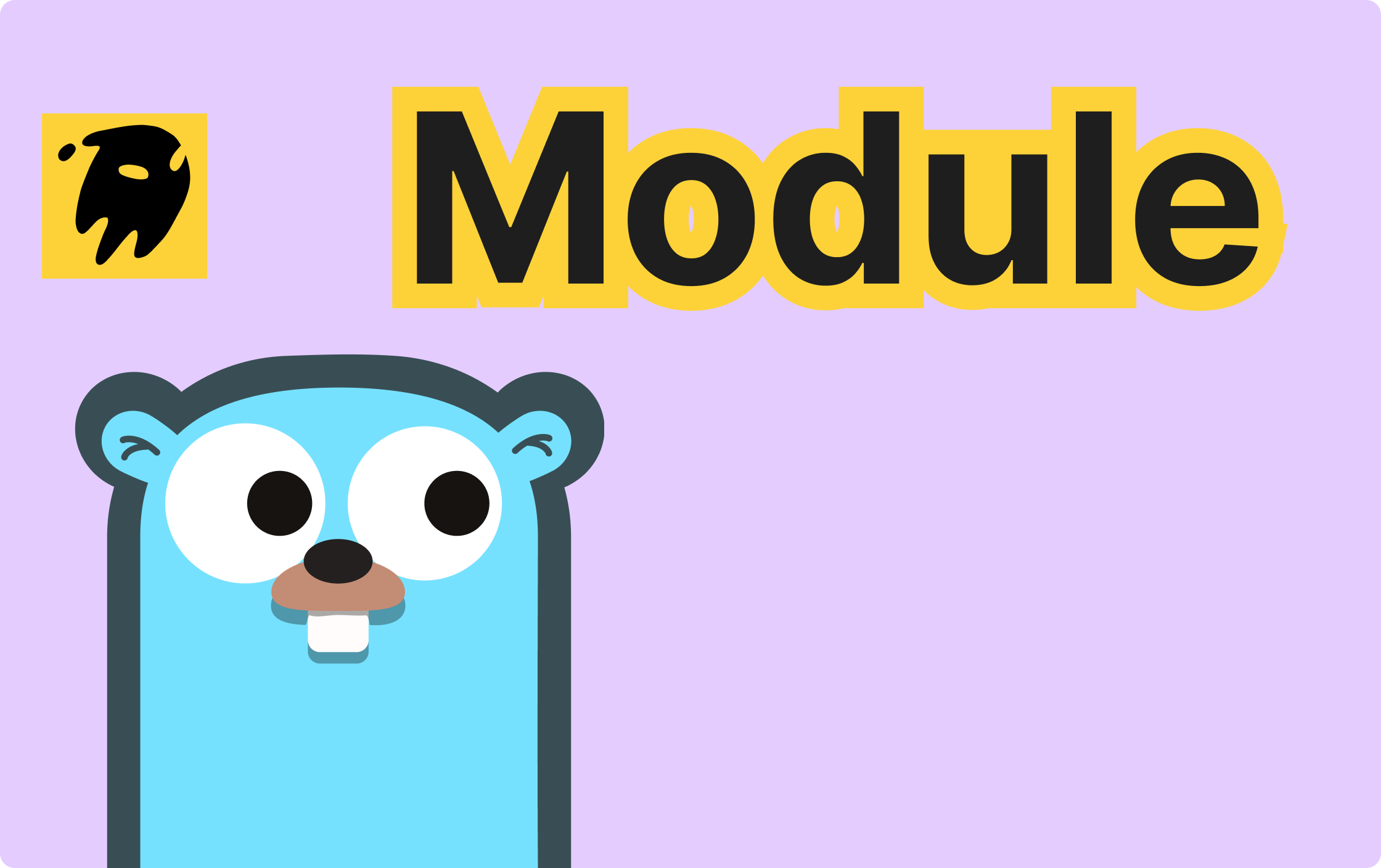
In-Depth Analysis of Go Module Principles: The Core Mechanism of Modern Go Dependency Management
I. Introduction
Go has become a mainstream programming language in cloud computing and microservices due to its high performance and concise syntax. As project scales expand, traditional dependency management solutions (GOPATH + Vendor) increasingly reveal flaws in version conflicts, collaboration efficiency, and build reliability. Introduced in Go 1.11, Go Module—the official dependency management solution—restructures Go project dependency management through modular design, semantic versioning, and automated dependency resolution. This article dissects how Go Module achieves efficient and reliable dependency management from three dimensions: design principles, core components, and operational mechanisms.
II. From GOPATH to Module: The Evolution of Dependency Management
2.1 Limitations of the GOPATH Era
- Single Workspace Model: All project dependencies are forcibly stored in the
GOPATH/src
directory, leading to unsolvable version conflicts between projects (e.g., Project A requires Library X@v1.0, while Project B requires Library X@v2.0). - High Collaboration Costs: Team members must manually synchronize dependency versions, lacking a declarative recording method, making it difficult to ensure consistency in the build environment.
- Drawbacks of the Vendor Transition Solution: Isolation is achieved by copying dependencies into the project, but this causes code repository bloat (redundant storage) and cumbersome version updates (manual maintenance).
2.2 Revolutionary Breakthroughs of Go Module (Go 1.11+)
- Liberation from GOPATH Restrictions: Projects can reside in any directory. Dependency management is module-centric, with dependency relationships explicitly declared in the
go.mod
file. - Semantic Versioning: Adopts the SemVer specification, with version formats like
vX.Y.Z
(major.minor.patch), clearly defining version compatibility. - Automated Toolchain: The
go mod
command set (e.g.,go mod tidy
/go mod vendor
) automates the entire process of dependency resolution, download, and update.
III. Core Components and Working Principles of Go Module
3.1 The Cornerstone of Module Declaration: The go.mod File
The go.mod
file in the project root directory is the core descriptor of a module, containing three key pieces of information:
module example.com/myproject // Module path (usually the code repository address) go 1.18 // Minimum compatible Go version for the project require ( fmtv v1.2.3 // Explicit dependency declaration (module name + version number) netv v2.0.0 // Explicit path declaration for different major versions (e.g., v2 version path is module@v2) ) replace example.com/netv v2.0.0 => ../local-netv // Local replacement (for development and debugging) exclude example.com/badv v1.0.0 // Exclude specific version dependencies
3.2 Core Logic of Dependency Resolution
3.2.1 Minimal Version Selection (MVS) Algorithm
When multiple dependencies point to different versions of the same module, Go selects the lowest version that satisfies all dependency constraints. For example:
- Project A depends on Library X@v1.2.0
- Library X@v1.2.0 depends on Library Y@v1.1.0
- Project A directly depends on Library Y@v1.2.0
- Final Resolution Result: Library Y@v1.2.0 (the smallest compatible version that satisfies all dependencies)
3.2.2 Dependency Graph Construction Process
- Initialization: Run
go mod init
to generate an initialgo.mod
, declaring the module path and Go version. - Dependency Discovery: Identify undeclared dependencies via
import
statements during compilation and automatically add them togo.mod
. - Version Resolution: Build a conflict-free dependency version tree based on
require
declarations and the MVS algorithm. - Download and Verification: Pull dependencies from module proxies and verify checksums against
go.sum
records (to prevent tampering).
3.3 Dependency Integrity Assurance: go.sum File and Checksum Database
- Role of go.sum: Records the module path, version, and SHA-256 checksum of all dependencies, ensuring completely consistent dependency content for every build.
- Checksum Database: A public service maintained by the Go team (
sum.golang.org
) that stores checksums of globally public modules to prevent supply chain attacks (e.g., poisoned dependencies).
3.4 Performance Optimization: Module Proxies and Local Caching
- Module Proxy (GOPROXY): Accelerates downloads and addresses network restrictions via intermediate servers caching dependencies (e.g., the official proxy
https://proxy.golang.org
or Alibaba Cloud proxyhttps://mirrors.aliyun.com/goproxy/
). - Local Cache Path: Downloaded dependencies are stored in
$GOPATH/pkg/mod
(default), supporting cross-project sharing to reduce redundant download overhead.
IV. Key Mechanisms and Practices of Dependency Management
4.1 Best Practices for Version Control
- Upgrading Dependencies:
go get -u
: Upgrades all dependencies to the latest compatible versions (following SemVer backward compatibility rules).go get example.com/lib@v2.1.0
: Upgrades to a specified version.
- Version Locking: Maintain consistency between
go.mod
andgo.sum
viago mod tidy
to ensure reproducible builds.
4.2 Private Module Management Solutions
- Environment Variable Configuration:
export GOPRIVATE="git.example.com/*,example.com/internal/*" # Declare private module paths export GOPROXY="https://proxy.example.com,direct" # Prioritize private proxies
- Authentication Methods: Access private repositories via Git credentials (SSH keys, Tokens) or proxy server authentication.
4.3 Dependency Replacement and Debugging
During development, use the replace
directive in go.mod
to point remote dependencies to local paths:
replace example.com/lib v1.0.0 => ../local-lib # Temporarily use local code for debugging
V. Comparison with Dependency Management in Other Languages
Feature | Go Module | Python Pip | Java Maven |
---|---|---|---|
Dependency Declaration | Explicit version declaration in go.mod | Version ranges in requirements.txt | Version/range in pom.xml |
Version Locking | Automatically generated checksums in go.sum | Pipfile.lock (requires tooling) | Fixed versions in pom.xml |
Dependency Storage | Global cache (GOPATH/pkg ) | Project virtual environment isolation | Local repository (~/.m2 ) |
Proxy Support | Built-in GOPROXY variable | Configured in pip.conf | Configured in settings.xml |
Private Module Support | Environment variables + path matching | Repository URL authentication | Repository configuration + authentication |
VI. Conclusion: The Design Philosophy of Go Module
Go Module establishes an efficient and reliable dependency management system in modern programming languages through four pillars: explicit declaration (go.mod
), automatic resolution (MVS algorithm), security verification (checksums), and performance optimization (proxy caching). Its core design philosophy can be summarized as:
- Minimized Human Intervention: The toolchain automatically handles dependency resolution and updates, reducing developer cognitive load.
- Reproducible Builds: Ensures consistent build results across environments via version locking and checksum mechanisms.
- Compatible Evolution: Supports smooth migration from GOPATH while providing modular support for large-scale microservices architectures.
Mastering the principles and practices of Go Module is a must for deepening Go development expertise and serves as the core foundation for building robust and maintainable modern Go projects.
Leapcell: The Best of Serverless Web Hosting
Finally, we recommend the best platform for deploying Go services: Leapcell
🚀 Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
🌍 Deploy Unlimited Projects for Free
Only pay for what you use—no requests, no charges.
⚡ Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
🔹 Follow us on Twitter: @LeapcellHQ