Getting Started with NestJS: Build Skills Step by Step
Daniel Hayes
Full-Stack Engineer · Leapcell
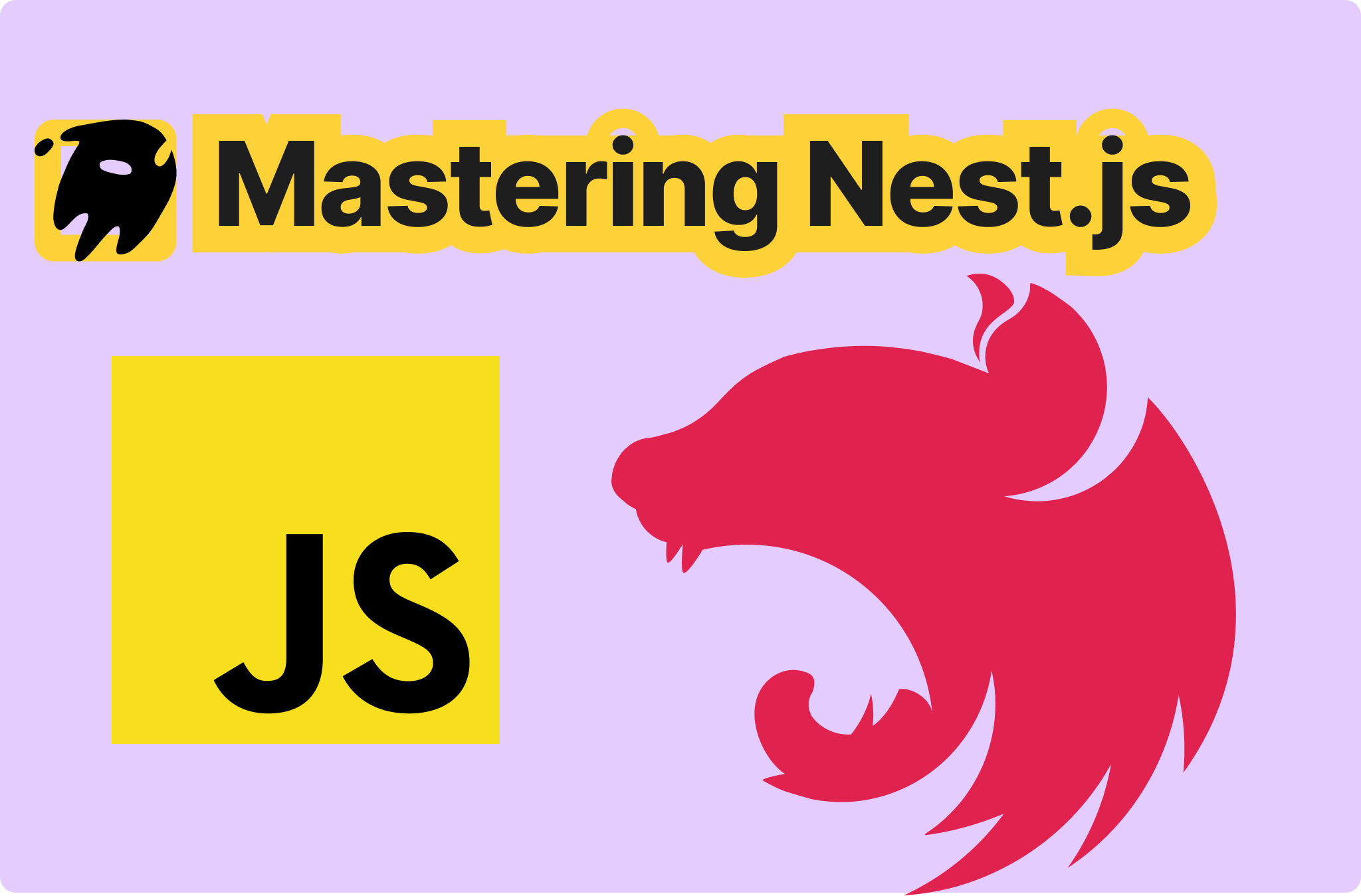
In the field of Node.js development, there are a wide variety of technical solutions for building web applications. At the beginning of development, we can choose to use the most basic native HTTP module. However, as the scale of the project continues to expand, the handwritten routing and business logic will become increasingly difficult to maintain. At this time, Express, with its simple design, simplifies the request handling and routing management processes, greatly improving development efficiency. In recent years, with the development of front-end frameworks, Next.js, as a full-stack framework based on React, has emerged. It has unique advantages in server-side rendering and static website generation. And when the complexity of the project climbs, especially in the context of enterprise-level application development, the flexibility of Express may become a hindrance. NestJS, as a modern and modular framework, provides a better solution for the development of complex projects with its powerful architectural design capabilities. This article will start from the native HTTP development of Node.js, gradually analyze the significant advantages of NestJS when dealing with complex projects, and conduct a comprehensive comparison with Express and Next.js.
Starting from the Basics: Using the Native HTTP Module of Node.js
Using the http
module that comes with Node.js, we can build a simple server:
const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, World!\n'); }); server.listen(3000, () => { console.log('Server running at http://127.0.0.1:3000/'); });
The above code creates a simple HTTP server that can respond to any request and return the string "Hello, World!". Although this method is suitable for beginners to understand the working principle of HTTP requests, in actual projects, especially when dealing with multiple routes or complex requests, it will become extremely cumbersome. Take handling multiple routes as an example:
const server = http.createServer((req, res) => { if (req.url === '/hello') { res.end('Hello Route!'); } else if (req.url === '/bye') { res.end('Goodbye Route!'); } else { res.statusCode = 404; res.end('Not Found'); } });
There are two obvious problems with the above code: every time a new route is added, the request path needs to be manually checked; there is a lack of a middleware mechanism, making it difficult to handle common requirements such as authentication and logging. Given these limitations, developers usually choose to introduce lightweight frameworks, and Express is one of the best choices.
Moving towards Simplification: Using Express to Improve the Code Structure
Express encapsulates the HTTP request handling process and provides a simple API to create routes and use middleware, greatly improving development efficiency. The code to achieve the same functionality using Express is as follows:
const express = require('express'); const app = express(); app.get('/hello', (req, res) => { res.send('Hello Route!'); }); app.get('/bye', (req, res) => { res.send('Goodbye Route!'); }); app.listen(3000, () => { console.log('Express server running on http://127.0.0.1:3000/'); });
Compared with the native HTTP module, the advantages of Express are obvious: route definition is more concise, there is no need to manually judge the URL and set the status code; the introduction of middleware makes it more convenient to handle complex business logic, such as authentication, logging, etc.
However, as the scale of the project continues to expand, the flexibility of Express will also bring some challenges. On the one hand, the code organization will become complex. When the project contains dozens or even hundreds of routes and business logic, if all the logic is piled up in a few files, code maintenance will become extremely difficult. Even if the routes are split into multiple files, as the business complexity increases, the route files may still become bloated. Take the user management route as an example:
const express = require('express'); const app = express(); // User management routes app.get('/users', (req, res) => { res.send('List of users'); }); app.post('/users', (req, res) => { res.send('Create a new user'); }); app.put('/users/:id', (req, res) => { res.send('Update user'); }); app.delete('/users/:id', (req, res) => { res.send('Delete user'); });
When more functions need to be added, this simple structure will be difficult to handle, and developers have to manually split and organize the files, resulting in a chaotic code structure. On the other hand, Express lacks a mandatory architectural specification, and each developer can organize the project code in their own way. This flexibility may be useful in small projects, but in large team collaborations, it will bring many problems: the code structure is inconsistent, and the code styles of different developers vary greatly; the code is difficult to maintain and expand, and modifying one function may affect other parts.
A New Force in the Web Ecosystem of Lightweight Frameworks: Next.js
Next.js, as a full-stack framework based on React, has opened up a new direction in the field of web development. It natively supports server-side rendering (SSR) and static website generation (SSG), which makes the developed applications perform well in terms of performance and search engine optimization (SEO). The routing system of Next.js is based on the file system, and routes can be easily defined by creating page files, greatly simplifying route management. At the same time, Next.js also provides a rich API for handling common tasks such as data fetching and state management, making the development process more efficient. However, Next.js focuses more on front-end rendering and full-stack application development, and it is relatively lacking in the in-depth support for back-end service architecture design and enterprise-level application development.
From Simple to Excellent: NestJS Builds a Better Architecture for Complex Applications
NestJS provides a modular and scalable architecture to help developers manage the project structure more efficiently. Different from the flexibility of Express, NestJS enforces a layered architecture, making the code clearer and easier to maintain.
The modular design of NestJS allows the project to be divided into multiple independent modules, each module focusing on a specific function; the separation of controllers and services transfers the business logic to the service layer, and the controllers are only responsible for handling requests; the introduction of the dependency injection mechanism makes the dependency relationship between modules clearer and the code coupling lower.
Taking the refactoring of the user management example as an example, the code structure of NestJS is as follows:
Controller (users.controller.ts)
import { Controller, Get, Post, Put, Delete, Param, Body } from '@nestjs/common'; import { UsersService } from './users.service'; import { CreateUserDto, UpdateUserDto } from './dto'; @Controller('users') export class UsersController { constructor(private readonly usersService: UsersService) {} @Get() getUsers() { return this.usersService.getAllUsers(); } @Post() createUser(@Body() createUserDto: CreateUserDto) { return this.usersService.createUser(createUserDto); } @Put(':id') updateUser(@Param('id') id: string, @Body() updateUserDto: UpdateUserDto) { return this.usersService.updateUser(id, updateUserDto); } @Delete(':id') deleteUser(@Param('id') id: string) { return this.usersService.deleteUser(id); } }
Service (users.service.ts)
import { Injectable } from '@nestjs/common'; import { CreateUserDto, UpdateUserDto } from './dto'; @Injectable() export class UsersService { private users = []; getAllUsers() { return 'List of users'; } createUser(createUserDto: CreateUserDto) { return 'Create a new user'; } updateUser(id: string, updateUserDto: UpdateUserDto) { return 'Update user'; } deleteUser(id: string) { return 'Delete user'; } }
In NestJS, the controller and business logic are clearly separated, and each module has a clear responsibility. This structure ensures that the readability and maintainability of the code can still be guaranteed when the project scale expands.
Comparison Table of NestJS, Next.js, and Express
Feature | NestJS | Next.js | Express |
---|---|---|---|
Architectural Design | Modular, layered architecture, mandatory compliance with specifications | Based on React, focusing on front-end rendering and full-stack development | High flexibility, lack of mandatory architectural specifications |
Route Management | Define routes through decorators | Route system based on the file system | Define routes through a simple API |
Code Organization | Separation of modules, controllers, and services, clear code structure | Relatively loose organization of front-end and back-end code | Increasing difficulty in code organization as the project scale grows |
Applicable Scenarios | Enterprise-level application development, high requirements for back-end architecture | Full-stack application development, focusing on front-end rendering and SEO | Quickly building lightweight web applications |
Dependency Injection | Supported | Not natively supported | Not natively supported |
Conclusion
From the native HTTP module of Node.js to Express, and then to NestJS and Next.js, we have witnessed the continuous evolution of web application development technologies. With its powerful architectural design capabilities, NestJS provides a structured solution for enterprise-level application development, making it an ideal choice for dealing with complex projects. Next.js, on the other hand, shows unique advantages in the fields of full-stack applications and front-end rendering. Although Express has certain limitations in large projects, it still has irreplaceable value when quickly building lightweight web applications. Developers should choose the most suitable technical solution according to the specific requirements of the project. Subsequent articles will delve into the core concepts and practical skills of NestJS to help developers quickly master this powerful framework.
Leapcell: The Best of Serverless Web Hosting
Finally, I recommend a platform that is most suitable for deploying Node.js services: Leapcell
🚀 Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
🌍 Deploy Unlimited Projects for Free
Only pay for what you use—no requests, no charges.
⚡ Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
🔹 Follow us on Twitter: @LeapcellHQ