How to Read .aspx Files in Node.js
Daniel Hayes
Full-Stack Engineer · Leapcell
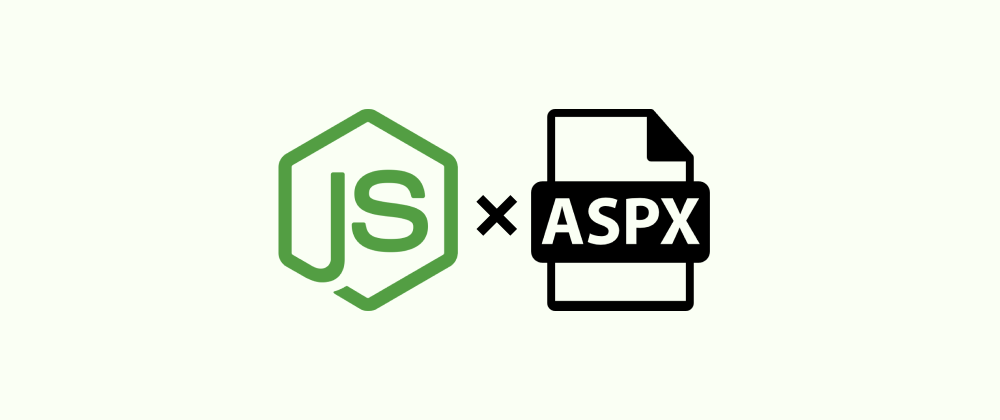
Reading and processing .aspx
files in a Node.js environment can be challenging due to the fundamental differences between Node.js and ASP.NET frameworks. .aspx
files are designed to be executed within an ASP.NET environment, where they are processed on the server side to generate dynamic HTML content. Node.js, on the other hand, is a JavaScript runtime that operates outside of the .NET ecosystem.
Key Takeaways
.aspx
files can be read in Node.js as static HTML files.- Server-side logic in
.aspx
requires accessing rendered HTML or .NET integration. - Use libraries like
cheerio
oraxios
for parsing and fetching content.
Steps
If your goal is to parse or extract information from an .aspx
file using Node.js, you can treat it as a standard HTML file, provided it contains static HTML content. Here's how you can do it:
-
Read the
.aspx
File: Use Node.js's built-infs
module to read the file's content.const fs = require('fs'); const filePath = 'path/to/your/file.aspx'; fs.readFile(filePath, 'utf8', (err, data) => { if (err) { console.error('Error reading the file:', err); return; } // 'data' contains the content of the .aspx file console.log(data); });
-
Parse the HTML Content: If the
.aspx
file contains HTML that you wish to manipulate or extract data from, you can use libraries likecheerio
to parse and traverse the HTML.First, install the
cheerio
package:npm install cheerio
Then, use it in your script:
const cheerio = require('cheerio'); fs.readFile(filePath, 'utf8', (err, data) => { if (err) { console.error('Error reading the file:', err); return; } const $ = cheerio.load(data); // Example: Extract the title of the HTML page const title = $('title').text(); console.log('Page Title:', title); });
However, if the .aspx
file relies on server-side code (such as C#) to generate dynamic content, simply reading and parsing the file in Node.js will not execute that server-side code. In such cases, you have a couple of options:
-
Access the Rendered HTML via HTTP: If the
.aspx
page is hosted on a server, you can make an HTTP request to the URL of the page to retrieve the rendered HTML. This way, you get the final output after all server-side processing has been done.const axios = require('axios'); const url = 'http://example.com/page.aspx'; axios.get(url) .then(response => { const htmlContent = response.data; // You can now parse 'htmlContent' using cheerio or any other method console.log(htmlContent); }) .catch(error => { console.error('Error fetching the page:', error); });
-
Integrate .NET with Node.js: If you need to execute server-side code within the
.aspx
file, consider using tools that allow interoperability between Node.js and .NET. One such tool isEdge.js
, which enables running .NET and Node.js code in the same process. This approach is more complex and is typically used when there's a need to integrate functionalities from both environments. (GitHub)
FAQs
No, Node.js cannot directly execute server-side .aspx
logic; use a .NET runtime or access via HTTP.
Use the fs
module to read the file and cheerio
for HTML parsing.
Use Edge.js
to run .NET code within a Node.js process.
Conclusion
In summary, while you can read and parse .aspx
files in Node.js as static HTML, executing server-side logic contained within them requires more advanced setups, such as accessing the rendered HTML via HTTP requests or integrating Node.js with .NET using tools like Edge.js
.
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ