How to Get a Timestamp Using Moment.js in Node.js
James Reed
Infrastructure Engineer · Leapcell
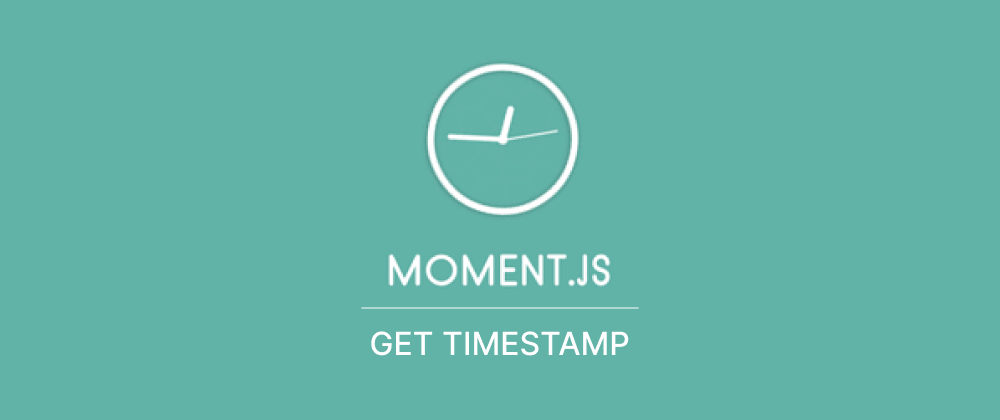
Moment.js is a popular JavaScript library for working with dates and times. If you're working in a Node.js environment and need to get timestamps, Moment.js makes it easy. This tutorial will walk you through the steps to install Moment.js, use it to get the current timestamp, and format timestamps according to your requirements.
Key Takeaways
- Moment.js simplifies timestamp retrieval and formatting in Node.js.
- It supports Unix timestamps in seconds and milliseconds.
- Moment.js offers extensive date manipulation and formatting features.
Step 1: Install Moment.js
First, ensure you have Node.js installed on your machine. If not, you can download and install it from Node.js official website.
Then, install Moment.js using npm by running the following command in your terminal:
npm install moment
Step 2: Import Moment.js in Your Code
To use Moment.js, you need to import it into your Node.js application. Create a file (e.g., app.js
) and add the following line at the beginning:
const moment = require('moment');
Step 3: Get the Current Timestamp
To get the current timestamp, you can use the moment().unix()
method. This will give you the timestamp in seconds since the Unix epoch (January 1, 1970).
const moment = require('moment'); // Get the current timestamp in seconds const timestampInSeconds = moment().unix(); console.log('Timestamp in seconds:', timestampInSeconds);
If you need the timestamp in milliseconds, use the moment().valueOf()
method:
const timestampInMilliseconds = moment().valueOf(); console.log('Timestamp in milliseconds:', timestampInMilliseconds);
Step 4: Format Timestamps
Moment.js allows you to format timestamps into human-readable strings or convert them into different time zones. For example:
const formattedDate = moment().format('YYYY-MM-DD HH:mm:ss'); console.log('Formatted Date:', formattedDate);
You can also parse a timestamp and format it:
const unixTimestamp = 1672531200; // Example timestamp const formattedFromTimestamp = moment.unix(unixTimestamp).format('YYYY-MM-DD HH:mm:ss'); console.log('Formatted Date from Timestamp:', formattedFromTimestamp);
Step 5: Additional Features of Moment.js
Moment.js provides a wide range of features beyond basic timestamp handling:
- Time Zone Support: Use the
moment-timezone
extension to handle time zones. - Date Math: Add or subtract days, months, or years easily.
- Relative Time: Display time differences like "2 hours ago" or "in 3 days."
For example, to add 7 days to the current date:
const nextWeek = moment().add(7, 'days').format('YYYY-MM-DD'); console.log('Date Next Week:', nextWeek);
Step 6: Consider Alternatives
Moment.js is a powerful library but is considered "legacy" as of now. For new projects, you might consider modern alternatives like Day.js or Luxon. However, Moment.js remains widely used in existing projects and provides robust functionality for handling timestamps.
FAQs
Use moment().valueOf()
to get the current timestamp in milliseconds.
Yes, use moment().format('YYYY-MM-DD HH:mm:ss')
to format timestamps.
No, Moment.js is legacy; consider using alternatives like Day.js or Luxon for new projects.
Conclusion
Moment.js simplifies working with dates and timestamps in Node.js. With just a few lines of code, you can get timestamps, format dates, and perform complex date manipulations. Follow this tutorial to incorporate Moment.js into your Node.js applications and handle dates and times effortlessly!
We are Leapcell, your top choice for hosting Node.js projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ