How to Get the Current Working Directory in Python
Grace Collins
Solutions Engineer · Leapcell
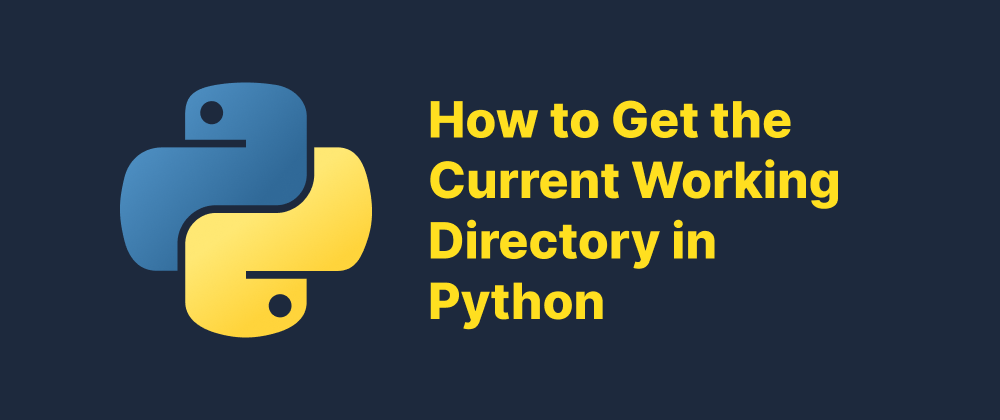
Key Takeaways
- You can retrieve the current working directory using
os.getcwd()
orPath.cwd()
. pathlib.Path.cwd()
is the modern, recommended approach for path handling in Python.- Understanding the working directory is essential when dealing with relative file paths.
When working with file systems in Python, it’s often necessary to know the current working directory (CWD)—the folder in which your Python script is running or where relative file operations are based. Python provides simple ways to access this information using the built-in os
and pathlib
modules. In this article, we’ll walk through multiple approaches to retrieve the current working directory.
Using the os
Module
The os
module provides a straightforward method called os.getcwd()
to get the current working directory.
import os cwd = os.getcwd() print("Current Working Directory:", cwd)
This will output the absolute path to the current working directory. For example:
Current Working Directory: /Users/yourname/projects/myapp
Using the pathlib
Module (Recommended in Modern Python)
Starting from Python 3.4, the pathlib
module offers an object-oriented interface for file system paths. It’s now the preferred way to handle filesystem paths in Python.
from pathlib import Path cwd = Path.cwd() print("Current Working Directory:", cwd)
This achieves the same result but returns a Path
object, which is more flexible when performing path manipulations.
Comparing os.getcwd()
vs Path.cwd()
Method | Return Type | Python Version | Notes |
---|---|---|---|
os.getcwd() | str | All versions | Simpler, widely supported |
Path.cwd() | Path object | 3.4+ | Recommended for new codebases |
Practical Tips
- If you want to change the working directory, you can use
os.chdir(path)
. - Always check the current working directory before opening files using relative paths.
- Use
Path
methods like.joinpath()
and.resolve()
for safer path construction.
Conclusion
Whether you're writing scripts or building larger applications, knowing how to get the current working directory is a key skill. While both os.getcwd()
and Path.cwd()
are valid, using pathlib
offers more robust and modern path handling capabilities.
FAQs
os.getcwd()
returns a string, while Path.cwd()
returns a Path
object that supports path operations.
Yes, use os.chdir(path)
to change the working directory.
Use pathlib
in modern Python (3.4+) for cleaner, object-oriented path manipulations.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ