How to Convert JSON to TypeScript Interfaces
Daniel Hayes
Full-Stack Engineer · Leapcell
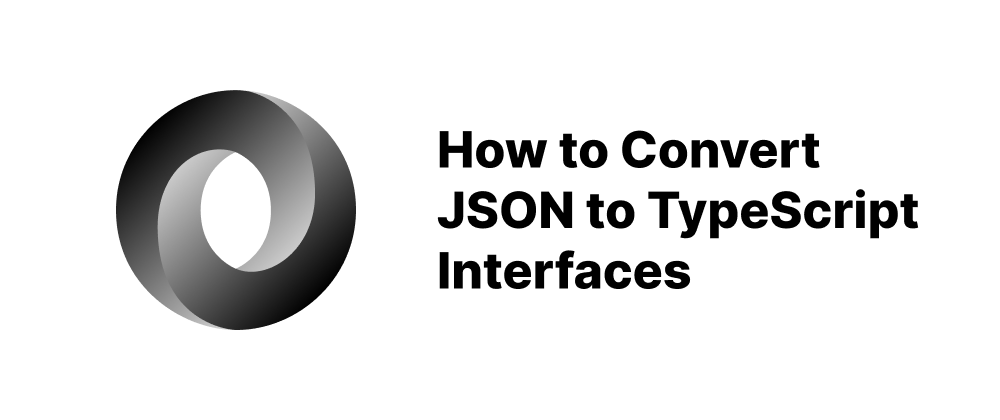
Key Takeaways
- JSON can be manually or automatically converted into TypeScript interfaces.
- Tools like Quicktype and VS Code extensions simplify the conversion process.
- Runtime validation ensures JSON data matches expected structures.
Converting JSON data into TypeScript interfaces enhances type safety and developer productivity by ensuring that your data structures are well-defined and consistent. This article explores various methods and tools to efficiently transform JSON into TypeScript interfaces.
Manual Conversion
For simple JSON structures, manual conversion is straightforward. Consider the following JSON object:
{ "name": "Alice", "age": 30, "isSubscriber": true }
You can define a corresponding TypeScript interface as follows:
interface User { name: string; age: number; isSubscriber: boolean; }
While manual conversion works for small and simple structures, it becomes cumbersome and error-prone with complex or deeply nested JSON data.
Online Tools
Quicktype
Quicktype is a powerful online tool that converts JSON into TypeScript interfaces, complete with optional runtime type-checking code. It supports various input formats, including JSON, JSON Schema, and GraphQL queries. You can use Quicktype directly in the browser or via the command line:
npm install -g quicktype quicktype -s json -o MyInterface.ts --lang ts myData.json
This command reads myData.json
and outputs a TypeScript file MyInterface.ts
with the generated interfaces.
Transform.tools
Transform.tools offers a user-friendly interface to convert JSON to TypeScript. Simply paste your JSON data, and it instantly generates the corresponding TypeScript interfaces. This tool is ideal for quick conversions without additional configurations.
Visual Studio Code Extensions
For developers using Visual Studio Code, several extensions facilitate the conversion of JSON to TypeScript interfaces directly within the editor.
JSON to TS
The JSON to TS extension allows you to convert JSON objects to TypeScript interfaces seamlessly. After installing the extension:
- Copy your JSON data to the clipboard.
- In VS Code, press
Shift + Ctrl + Alt + V
(Windows/Linux) orShift + Cmd + Alt + V
(macOS) to paste the JSON as TypeScript interfaces.
This extension supports features like array type merging, duplicate type prevention, and optional types.
Paste JSON as Code
The Paste JSON as Code extension, powered by Quicktype, enables you to paste JSON data as TypeScript interfaces with runtime validation. To use it:
- Copy your JSON data.
- In VS Code, open the Command Palette (
Ctrl + Shift + P
orCmd + Shift + P
). - Select "Paste JSON as Code" and choose TypeScript as the target language.
This extension is particularly useful for generating interfaces with built-in validation logic.
Runtime Validation
When working with JSON data from external sources, it's crucial to validate the data at runtime to prevent unexpected errors. Quicktype can generate TypeScript code with runtime validation functions. For example:
import { Convert, User } from "./user"; const json = '{"name": "Alice", "age": 30, "isSubscriber": true}'; try { const user = Convert.toUser(json); console.log(user); } catch (e) { console.error("Invalid JSON data", e); }
In this example, Convert.toUser
parses and validates the JSON string, throwing an error if the data doesn't match the expected structure.
Conclusion
Converting JSON to TypeScript interfaces is an essential practice for maintaining type safety and improving code quality in TypeScript projects. Depending on your workflow and project complexity, you can choose between manual conversion, online tools like Quicktype and Transform.tools, or integrate the process into your development environment using Visual Studio Code extensions. Incorporating runtime validation further enhances the robustness of your applications by ensuring that incoming JSON data adheres to the expected formats.
For a deeper understanding of TypeScript and its capabilities, consider exploring resources like the Ultimate TypeScript Handbook or the TypeScript Cookbook.
FAQs
To improve type safety and prevent runtime errors.
Quicktype is highly recommended for both browser and CLI use.
Yes, tools like Quicktype offer runtime validation functions.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ