Error Wrapping in Go: Enhancing Debugging and Maintainability
Daniel Hayes
Full-Stack Engineer · Leapcell
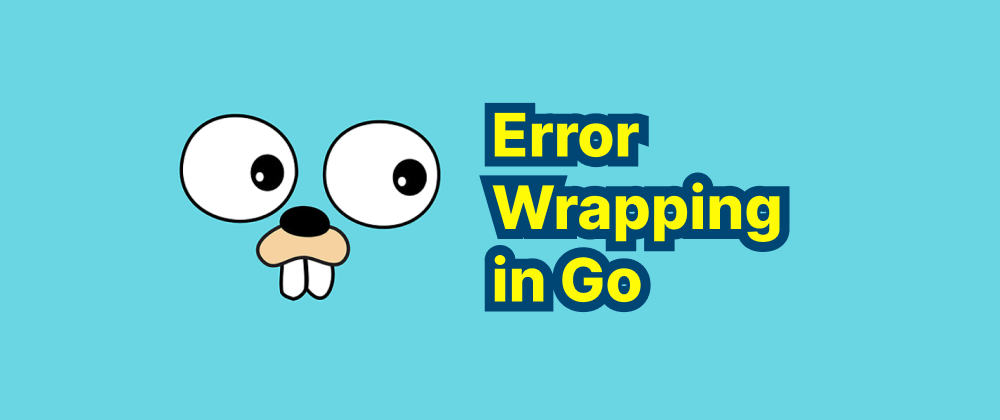
Key Takeaways
- Error wrapping preserves original context while adding meaningful debugging information.
- Go 1.13 introduced native error wrapping with
fmt.Errorf("%w", err)
, replacing external packages. - Use
errors.Is
,errors.As
, anderrors.Unwrap
to inspect and handle wrapped errors effectively.
Error handling is a crucial aspect of software development, enabling developers to create robust and maintainable applications. In Go, error wrapping is a technique that adds context to errors, facilitating easier debugging and improved code readability. This article explores the concept of error wrapping in Go, its evolution, and best practices for its implementation.
Understanding Error Wrapping
Error wrapping involves annotating an existing error with additional information without losing the original error context. This practice is particularly useful when errors propagate through multiple function calls, as it allows each function to add its own context, making it easier to trace the source of the problem.
Evolution of Error Wrapping in Go
Before Go 1.13
Prior to Go 1.13, the standard library lacked built-in support for error wrapping. Developers often relied on external packages, such as github.com/pkg/errors
, to enhance error handling capabilities. This package provided functions like Wrap
and Wrapf
to add context to errors along with stack traces.
import "github.com/pkg/errors" func readFile(filename string) ([]byte, error) { data, err := ioutil.ReadFile(filename) if err != nil { return nil, errors.Wrap(err, "failed to read file") } return data, nil }
In this example, if ioutil.ReadFile
returns an error, it is wrapped with a message providing additional context about the failure.
Introduction of fmt.Errorf
with %w
in Go 1.13
With the release of Go 1.13, the language introduced native support for error wrapping through the fmt.Errorf
function and the %w
verb. This enhancement allowed developers to wrap errors without external dependencies.
import ( "fmt" "io/ioutil" ) func readFile(filename string) ([]byte, error) { data, err := ioutil.ReadFile(filename) if err != nil { return nil, fmt.Errorf("failed to read file: %w", err) } return data, nil }
Here, the %w
verb in fmt.Errorf
wraps the original error, preserving its context.
Unwrapping and Inspecting Errors
Go's standard library provides functions like errors.Is
, errors.As
, and errors.Unwrap
to work with wrapped errors.
errors.Is
checks if an error matches a specific target error.
if errors.Is(err, os.ErrNotExist) { // handle file not found error }
errors.As
attempts to assign an error to a variable of a specific type.
var pathErr *os.PathError if errors.As(err, &pathErr) { // handle *os.PathError }
errors.Unwrap
retrieves the next error in the error chain.
unwrappedErr := errors.Unwrap(err)
These functions enable precise error handling and introspection.
Best Practices for Error Wrapping
- Add Contextual Information: When wrapping an error, include meaningful context to aid in debugging.
if err != nil { return fmt.Errorf("processing file %s: %w", filename, err) }
-
Avoid Over-Wrapping: Wrap errors judiciously to prevent obscuring the original error. Only add context when it provides additional value.
-
Use Sentinel Errors: Define sentinel errors for common failure scenarios and wrap them with specific context as needed.
var ErrNotFound = errors.New("not found") func findItem(id string) (*Item, error) { item, err := queryDatabase(id) if err != nil { return nil, fmt.Errorf("item %s: %w", id, ErrNotFound) } return item, nil }
- Leverage
errors.Is
anderrors.As
: Utilize these functions to check for specific error types or values, enhancing error handling flexibility.
if errors.Is(err, ErrNotFound) { // handle not found error }
Conclusion
Error wrapping is a powerful technique in Go that enhances error handling by preserving the original error context while adding valuable information. With the native support introduced in Go 1.13, developers can implement error wrapping more seamlessly, leading to more maintainable and debuggable codebases. By following best practices, such as adding meaningful context and using the appropriate error inspection functions, developers can effectively manage errors in their Go applications.
FAQs
It helps retain error context, making debugging easier and improving error handling clarity.
It provides built-in support via fmt.Errorf("%w", err)
, reducing dependency on third-party packages.
Use errors.Is
for checking specific error values and errors.As
for type assertion on error types.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ