How to Convert XML to JSON: A Practical Guide
Daniel Hayes
Full-Stack Engineer · Leapcell
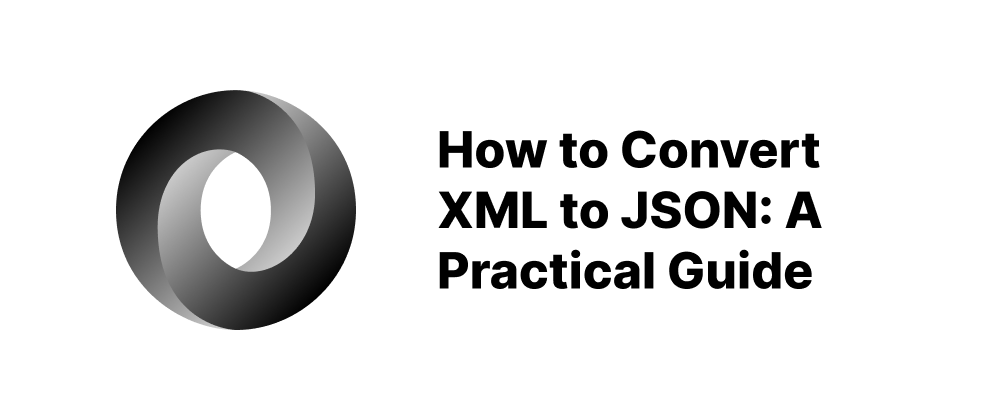
Key Takeaways
- JSON is more efficient and web-friendly than XML.
- Online tools offer fast XML-to-JSON conversion.
- Programming libraries enable automated, flexible conversions.
Converting XML (Extensible Markup Language) to JSON (JavaScript Object Notation) is a common task in modern web development and data integration. JSON is often preferred for its lightweight, readable structure and native compatibility with JavaScript-based applications. This guide outlines various methods to convert XML to JSON, catering to different needs and technical proficiencies.
Why Convert XML to JSON?
-
Web Compatibility: JSON integrates seamlessly with JavaScript, making it ideal for web applications.
-
Efficiency: JSON's concise syntax reduces data size, enhancing transmission speed and performance.
-
Ease of Use: JSON's straightforward structure simplifies parsing and data manipulation.
Method 1: Using Online Conversion Tools
For quick conversions without coding, online tools are highly effective.
Recommended Tools:
-
Code Beautify: Offers a user-friendly interface to paste XML and obtain formatted JSON instantly.
-
JSONFormatter.org: Allows XML input via text, file upload, or URL, providing formatted JSON output.
-
FreeFormatter.com: Converts XML to JSON while handling attributes and text nodes with customizable options.
-
Oxygen XML Editor: A comprehensive tool for XML editing and conversion, suitable for more complex tasks.
Steps:
-
Access the chosen tool's website.
-
Input your XML data by pasting it into the provided text area, uploading a file, or entering a URL.
-
Initiate the conversion process by clicking the appropriate button.
-
Review and download the resulting JSON output.
Method 2: Programmatic Conversion Using Python
For developers requiring automated or batch conversions, Python offers robust libraries.
Using xmltodict
Library:
import xmltodict import json # Read XML data from a file with open('data.xml', 'r') as xml_file: xml_data = xml_file.read() # Convert XML to a Python dictionary data_dict = xmltodict.parse(xml_data) # Convert dictionary to JSON json_data = json.dumps(data_dict, indent=4) # Write JSON data to a file with open('data.json', 'w') as json_file: json_file.write(json_data)
This script reads XML data, parses it into a Python dictionary, converts it to JSON, and writes the output to a file.
Method 3: Conversion Using Java
Java developers can utilize libraries like org.json
to perform XML to JSON conversions.
Using org.json
Library:
import org.json.JSONObject; import org.json.XML; public class XmlToJsonConverter { public static void main(String[] args) { String xml = "<note><to>User</to><from>Admin</from><message>Hello</message></note>"; JSONObject json = XML.toJSONObject(xml); System.out.println(json.toString(4)); } }
This Java program converts a simple XML string into a formatted JSON output.
Considerations During Conversion
-
Attributes Handling: XML attributes may be represented differently in JSON. Some tools prefix attribute names with symbols like
@
to distinguish them. -
Arrays and Repeated Elements: Repeated XML elements are typically converted into JSON arrays.
-
Text Content: Mixed content (elements containing both text and child elements) requires careful handling to preserve data integrity.
-
Data Types: XML does not inherently define data types, so type inference during conversion may not always be accurate.
Conclusion
Converting XML to JSON is essential for integrating legacy systems with modern web applications. Depending on your requirements, you can choose between online tools for quick conversions or programming libraries for more control and automation. Always consider the structure and complexity of your XML data to select the most appropriate conversion method.
FAQs
JSON is more compact and integrates better with modern web apps.
Yes, online tools like Code Beautify or JSONFormatter allow no-code conversions.
Python (xmltodict
) and Java (org.json
) are both effective choices.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ